UIManager resources to tweak the look of applications
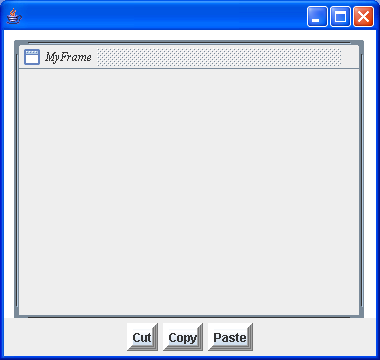
//An example of using UIManager resources to tweak the look of applications.
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.Font;
import javax.swing.BorderFactory;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JDesktopPane;
import javax.swing.JFrame;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.UIManager;
import javax.swing.border.Border;
import javax.swing.border.CompoundBorder;
public class ResourceModExample {
public static void main(String[] args) {
Border border = BorderFactory.createRaisedBevelBorder();
Border tripleBorder = new CompoundBorder(new CompoundBorder(border,border), border);
UIManager.put("Button.border", tripleBorder);
UIManager.put("InternalFrame.closeIcon", new ImageIcon("close.gif"));
UIManager.put("InternalFrame.iconizeIcon", new ImageIcon("iconify.gif"));
UIManager.put("InternalFrame.maximizeIcon", new ImageIcon("maximize.gif"));
UIManager.put("InternalFrame.altMaximizeIcon", new ImageIcon("altMax.gif"));
UIManager.put("InternalFrame.titleFont", new Font("Serif", Font.ITALIC,12));
UIManager.put("ScrollBar.width", new Integer(30));
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container c = f.getContentPane();
JDesktopPane desk = new JDesktopPane();
c.add(desk, BorderLayout.CENTER);
JButton cut = new JButton("Cut");
JButton copy = new JButton("Copy");
JButton paste = new JButton("Paste");
JPanel p = new JPanel(new FlowLayout());
p.add(cut);
p.add(copy);
p.add(paste);
c.add(p, BorderLayout.SOUTH);
JInternalFrame inf = new JInternalFrame("MyFrame", true, true, true,true);
JLabel l = new JLabel(new ImageIcon("luggage.jpeg"));
JScrollPane scroll = new JScrollPane(l);
inf.setContentPane(scroll);
inf.setBounds(10, 10, 350, 280);
desk.add(inf);
inf.setVisible(true);
f.setSize(380, 360);
f.setVisible(true);
}
}
Related examples in the same category