Implementing arithmetic and array functions
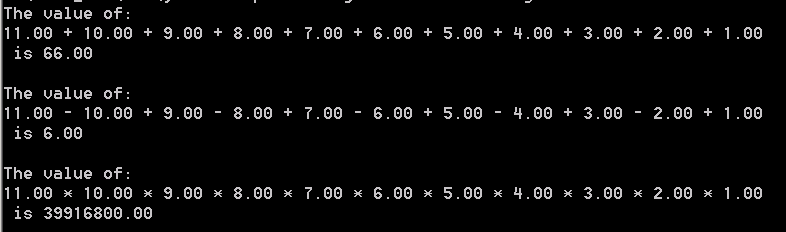
#include <stdio.h>
double add(double a, double b)
{
return a+b;
}
double subtract(double a, double b)
{
return a-b;
}
double multiply(double a, double b)
{
return a*b;
}
double array_op(double array[], int size, double (*pfun)(double,double))
{
double result = array[size-1];
int i = 0;
for(i = size-1 ; i>0 ; i--)
result = pfun(array[i-1], result);
return result;
}
int main()
{
double array[] = {11.0, 10.0, 9.0, 8.0, 7.0, 6.0, 5.0, 4.0, 3.0, 2.0, 1.0};
int i = 0;
int length = sizeof array/sizeof(double);
printf("The value of:\n");
for(i = 0 ; i< length ; i++)
{
printf("%.2f%s\n", array[i]);
}
printf(" is %.2lf\n", array_op(array,length,add));
printf("\nThe value of:\n");
for(i = 0 ; i< length ; i++)
{
printf("%.2lf%s\n", array[i]);
}
printf(" is %.2lf\n", array_op(array, length, subtract));
printf("\nThe value of:\n");
for(i = 0 ; i< length ; i++)
{
printf("%.2lf%s\n", array[i]);
}
printf(" is %.2lf\n", array_op(array, length, multiply));
}
Related examples in the same category