Class Hierarchy test
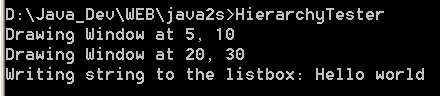
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
class Window
{
// constructor takes two integers to
// fix location on the console
public Window(int top, int left)
{
this.top = top;
this.left = left;
}
// simulates drawing the window
public void DrawWindow()
{
Console.WriteLine("Drawing Window at {0}, {1}",
top, left);
}
// these members are private and thus invisible
// to derived class methods; we'll examine this
// later in the chapter
private int top;
private int left;
}
// ListBox derives from Window
class ListBox : Window
{
// constructor adds a parameter
public ListBox(
int top,
int left,
string theContents):
base(top, left) // call base constructor
{
mListBoxContents = theContents;
}
// a new version (note keyword) because in the
// derived method we change the behavior
public new void DrawWindow()
{
base.DrawWindow(); // invoke the base method
Console.WriteLine ("Writing string to the listbox: {0}",
mListBoxContents);
}
private string mListBoxContents; // new member variable
}
public class HierarchyTester
{
public static void Main()
{
// create a base instance
Window w = new Window(5,10);
w.DrawWindow();
// create a derived instance
ListBox lb = new ListBox(20,30,"Hello world");
lb.DrawWindow();
}
}
Related examples in the same category