Demonstrate a destructor
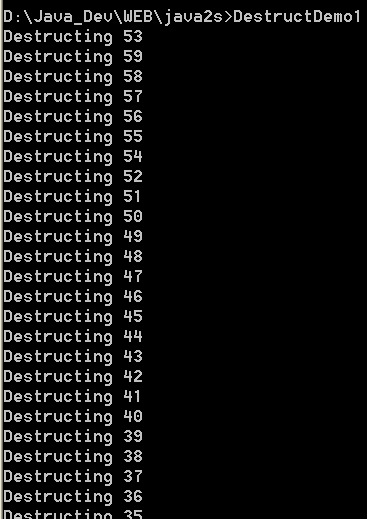
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate a destructor.
using System;
class Destruct {
public int x;
public Destruct(int i) {
x = i;
}
// called when object is recycled
~Destruct() {
Console.WriteLine("Destructing " + x);
}
// generates an object that is immediately destroyed
public void generator(int i) {
Destruct o = new Destruct(i);
}
}
public class DestructDemo1 {
public static void Main() {
int count;
Destruct ob = new Destruct(0);
/* Now, generate a large number of objects. At
some point, garbage collection will occur.
Note: you might need to increase the number
of objects generated in order to force
garbage collection. */
for(count=1; count < 100000; count++)
ob.generator(count);
Console.WriteLine("Done");
}
}
Related examples in the same category