Illustrates how to define a constructor
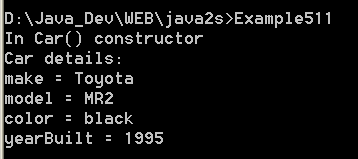
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example5_11.cs illustrates how to define a constructor
*/
// declare the Car class
class Car
{
// declare the fields
private string make;
private string model;
private string color;
private int yearBuilt;
// define the constructor
public Car(string make, string model, string color, int yearBuilt)
{
System.Console.WriteLine("In Car() constructor");
this.make = make;
this.model = model;
this.color = color;
this.yearBuilt = yearBuilt;
}
// define a method to display the fields
public void Display()
{
System.Console.WriteLine("Car details:");
System.Console.WriteLine("make = " + make);
System.Console.WriteLine("model = " + model);
System.Console.WriteLine("color = " + color);
System.Console.WriteLine("yearBuilt = " + yearBuilt);
}
}
public class Example5_11
{
public static void Main()
{
// create a Car object using the constructor
// defined in the class
Car myCar = new Car("Toyota", "MR2", "black", 1995);
// display the values for the Car object fields
myCar.Display();
}
}
Related examples in the same category