Method overloading test
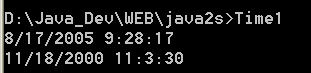
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
namespace MethodOverloading
{
public class Time1
{
// private member variables
private int Year;
private int Month;
private int Date;
private int Hour;
private int Minute;
private int Second;
// public accessor methods
public void DisplayCurrentTime()
{
System.Console.WriteLine("{0}/{1}/{2} {3}:{4}:{5}",
Month, Date, Year, Hour, Minute, Second);
}
// constructors
public Time1(System.DateTime dt)
{
Year = dt.Year;
Month = dt.Month;
Date = dt.Day;
Hour = dt.Hour;
Minute = dt.Minute;
Second = dt.Second;
}
public Time1(int Year, int Month, int Date,
int Hour, int Minute, int Second)
{
this.Year = Year;
this.Month = Month;
this.Date = Date;
this.Hour = Hour;
this.Minute = Minute;
this.Second = Second;
}
}
public class MethodOverloadingTester
{
public void Run()
{
System.DateTime currentTime = System.DateTime.Now;
Time1 time1 = new Time1(currentTime);
time1.DisplayCurrentTime();
Time1 time2 = new Time1(2000,11,18,11,03,30);
time2.DisplayCurrentTime();
}
static void Main()
{
MethodOverloadingTester t = new MethodOverloadingTester();
t.Run();
}
}
}
Related examples in the same category