Object Copy and Clone
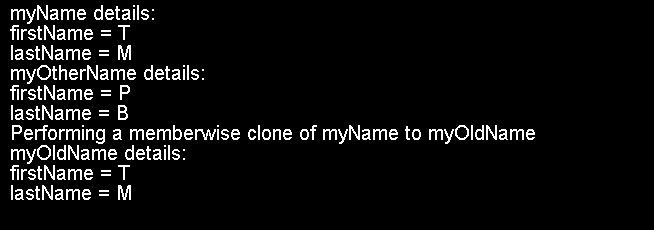
using System;
public class Name {
public string firstName;
public string lastName;
public Name(string firstName, string lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public void Display() {
Console.WriteLine("firstName = " + firstName);
Console.WriteLine("lastName = " + lastName);
}
public static Name Copy(Name car) {
return (Name) car.MemberwiseClone();
}
}
class Test {
public static void Main() {
Name myName = new Name("T", "M");
Name myOtherName = new Name("P", "B");
Console.WriteLine("myName details:");
myName.Display();
Console.WriteLine("myOtherName details:");
myOtherName.Display();
// perform a memberwise clone of myName using the Name.Copy() method
Console.WriteLine("Performing a memberwise clone of myName to myOldName");
Name myOldName = Name.Copy(myName);
Console.WriteLine("myOldName details:");
myOldName.Display();
}
}
Related examples in the same category