class declaration maintains the time in 24-hour format and ToString Method
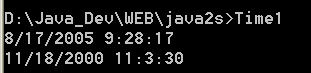
using System;
public class Time1
{
private int hour; // 0 - 23
private int minute; // 0 - 59
private int second; // 0 - 59
public void SetTime( int h, int m, int s )
{
hour = ( ( h >= 0 && h < 24 ) ? h : 0 );
minute = ( ( m >= 0 && m < 60 ) ? m : 0 );
second = ( ( s >= 0 && s < 60 ) ? s : 0 );
}
public string ToUniversalString()
{
return string.Format( "{0:D2}:{1:D2}:{2:D2}",
hour, minute, second );
}
public override string ToString()
{
return string.Format( "{0}:{1:D2}:{2:D2} {3}",
( ( hour == 0 || hour == 12 ) ? 12 : hour % 12 ),
minute, second, ( hour < 12 ? "AM" : "PM" ) );
}
}
public class Time1Test
{
public static void Main( string[] args )
{
Time1 time = new Time1();
Console.WriteLine( time.ToUniversalString() );
Console.WriteLine( time.ToString() );
time.SetTime( 13, 27, 6 );
Console.WriteLine( time.ToUniversalString() );
Console.WriteLine( time.ToString() );
time.SetTime( 99, 99, 99 );
Console.WriteLine( time.ToUniversalString() );
Console.WriteLine( time.ToString() );
}
}
Related examples in the same category