demonstrates using a structure to return a group of variables from a function
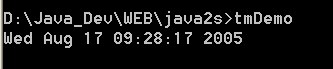
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// tm.cs - demonstrates using a structure to return a group of variables
// from a function
//
// Compile this program using the following command line:
// D:>csc tm.cs
//
namespace nsStructure
{
using System;
using System.Globalization;
public struct tm
{
public int tm_sec; // Seconds after the minute
public int tm_min; // Minutes after the hour
public int tm_hour; // Hours since midnight
public int tm_mday; // The day of the month
public int tm_mon; // The month (January = 0)
public int tm_year; // The year (00 = 1900)
public int tm_wday; // The day of the week (Sunday = 0)
public int tm_yday; // The day of the year (Jan. 1 = 1)
public int tm_isdst; // Flag to indicate if DST is in effect
}
public class tmDemo
{
static public void Main()
{
DateTime timeVal = DateTime.Now;
tm tmNow = LocalTime (timeVal);
string strTime = AscTime (tmNow);
Console.WriteLine (strTime);
}
static public tm LocalTime(DateTime tmVal)
{
tm time;
time.tm_sec = tmVal.Second;
time.tm_min = tmVal.Minute;
time.tm_hour = tmVal.Hour;
time.tm_mday = tmVal.Day;
time.tm_mon = tmVal.Month - 1;
time.tm_year = tmVal.Year - 1900;
time.tm_wday = (int) tmVal.DayOfWeek;
time.tm_yday = tmVal.DayOfYear;
TimeZone tz = TimeZone.CurrentTimeZone;
time.tm_isdst = tz.IsDaylightSavingTime (tmVal) == true ? 1 : 0;
return (time);
}
//
// Returns a string representing a time using UNIX format
static public string AscTime (tm time)
{
const string wDays = "SunMonTueWedThuFriSat";
const string months = "JanFebMarAprMayJunJulAugSepOctNovDec";
string strTime = String.Format ("{0} {1} {2,2:00} " +
"{3,2:00}:{4,2:00}:{5,2:00} {6}\n",
wDays.Substring (3 * time.tm_wday, 3),
months.Substring (3 * time.tm_mon, 3),
time.tm_mday, time.tm_hour,
time.tm_min, time.tm_sec, time.tm_year + 1900);
return (strTime);
}
}
}
Related examples in the same category