illustrates member hiding
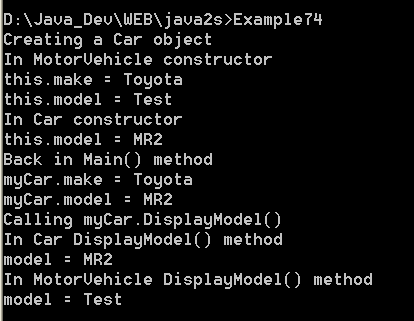
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_4.cs illustrates member hiding
*/
using System;
// declare the MotorVehicle class
class MotorVehicle
{
// declare the fields
public string make;
public string model;
// define a constructor
public MotorVehicle(string make, string model)
{
Console.WriteLine("In MotorVehicle constructor");
this.make = make;
this.model = model;
Console.WriteLine("this.make = " + this.make);
Console.WriteLine("this.model = " + this.model);
}
// define the DisplayModel() method
public void DisplayModel()
{
Console.WriteLine("In MotorVehicle DisplayModel() method");
Console.WriteLine("model = " + model);
}
}
// declare the Car class (derived from MotorVehicle)
class Car : MotorVehicle
{
// hide the base class model field
public new string model;
// define a constructor
public Car(string make, string model) :
base(make, "Test")
{
Console.WriteLine("In Car constructor");
this.model = model;
Console.WriteLine("this.model = " + this.model);
}
// hide the base class DisplayModel() method
public new void DisplayModel()
{
Console.WriteLine("In Car DisplayModel() method");
Console.WriteLine("model = " + model);
base.DisplayModel(); // calls DisplayModel() in the base class
}
}
public class Example7_4
{
public static void Main()
{
// create a Car object
Console.WriteLine("Creating a Car object");
Car myCar = new Car("Toyota", "MR2");
Console.WriteLine("Back in Main() method");
Console.WriteLine("myCar.make = " + myCar.make);
Console.WriteLine("myCar.model = " + myCar.model);
// call the Car object's DisplayModel() method
Console.WriteLine("Calling myCar.DisplayModel()");
myCar.DisplayModel();
}
}
Related examples in the same category