A simple version of the Quicksort
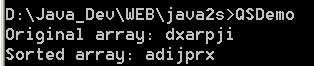
/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
// A simple version of the Quicksort.
using System;
class Quicksort {
// Set up a call to the actual Quicksort method.
public static void qsort(char[] items) {
qs(items, 0, items.Length-1);
}
// A recursive version of Quicksort for characters.
static void qs(char[] items, int left, int right)
{
int i, j;
char x, y;
i = left; j = right;
x = items[(left+right)/2];
do {
while((items[i] < x) && (i < right)) i++;
while((x < items[j]) && (j > left)) j--;
if(i <= j) {
y = items[i];
items[i] = items[j];
items[j] = y;
i++; j--;
}
} while(i <= j);
if(left < j) qs(items, left, j);
if(i < right) qs(items, i, right);
}
}
public class QSDemo {
public static void Main() {
char[] a = { 'd', 'x', 'a', 'r', 'p', 'j', 'i' };
int i;
Console.Write("Original array: ");
for(i=0; i < a.Length; i++)
Console.Write(a[i]);
Console.WriteLine();
// now, sort the array
Quicksort.qsort(a);
Console.Write("Sorted array: ");
for(i=0; i < a.Length; i++)
Console.Write(a[i]);
}
}
Related examples in the same category