Creates and accesses an array of classes. Implements the IComparable interface
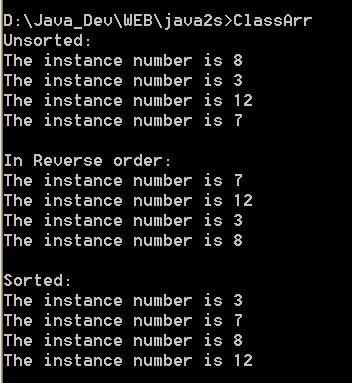
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// ClassArr.cs -- Creates and accesses an array of classes. Implements the
// IComparable interface.
//
// Compile this program with the folowing command line
// C:>csc ClassArr.cs
//
namespace nsForEach
{
using System;
public class ClassArr
{
static public void Main ()
{
clsElement [] Arr = new clsElement []
{
new clsElement (8), new clsElement (3),
new clsElement (12), new clsElement (7)
};
Console.WriteLine ("Unsorted:");
foreach (clsElement val in Arr)
{
Console.WriteLine ("The instance number is " + val.Instance);
}
Array.Reverse (Arr);
Console.WriteLine ("\r\nIn Reverse order:");
foreach (clsElement val in Arr)
{
Console.WriteLine ("The instance number is " + val.Instance);
}
Array.Sort (Arr);
Console.WriteLine ("\r\nSorted:");
foreach (clsElement val in Arr)
{
Console.WriteLine ("The instance number is " + val.Instance);
}
Console.WriteLine ();
}
}
class clsElement : IComparable
{
public clsElement (int instance)
{
m_Instance = instance;
}
private int m_Instance;
public int Instance
{
get{return (m_Instance);}
}
public int CompareTo (object o)
{
if (o.GetType() != this.GetType())
throw(new ArgumentException());
clsElement elem = (clsElement) o;
return (this.Instance - elem.Instance);
}
}
}
Related examples in the same category