illustrates the use of the Hashtable methods
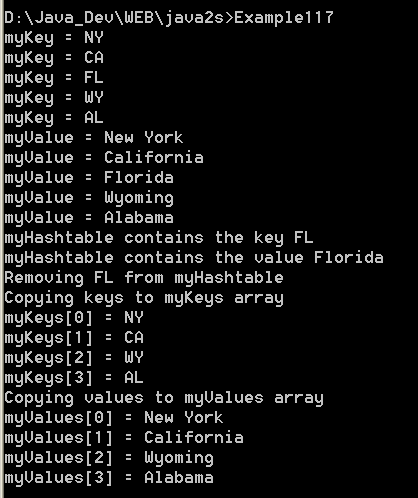
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example11_7.cs illustrates the use of the Hashtable methods
*/
using System;
using System.Collections;
public class Example11_7
{
public static void Main()
{
// create a Hashtable object
Hashtable myHashtable = new Hashtable();
// add elements containing US state abbreviations and state
// names to myHashtable using the Add() method
myHashtable.Add("AL", "Alabama");
myHashtable.Add("CA", "California");
myHashtable.Add("FL", "Florida");
myHashtable.Add("NY", "New York");
myHashtable.Add("WY", "Wyoming");
// display the keys for myHashtable using the Keys property
foreach (string myKey in myHashtable.Keys)
{
Console.WriteLine("myKey = " + myKey);
}
// display the values for myHashtable using the Values property
foreach(string myValue in myHashtable.Values)
{
Console.WriteLine("myValue = " + myValue);
}
// use the ContainsKey() method to check if myHashtable
// contains the key "FL"
if (myHashtable.ContainsKey("FL"))
{
Console.WriteLine("myHashtable contains the key FL");
}
// use the ContainsValue() method to check if myHashtable
// contains the value "Florida"
if (myHashtable.ContainsValue("Florida"))
{
Console.WriteLine("myHashtable contains the value Florida");
}
// use the Remove() method to remove FL from myHashtable
Console.WriteLine("Removing FL from myHashtable");
myHashtable.Remove("FL");
// get the number of elements in myHashtable using the Count
// property
int count = myHashtable.Count;
// copy the keys from myHashtable into an array using
// the CopyTo() method and then display the array contents
Console.WriteLine("Copying keys to myKeys array");
string[] myKeys = new string[count];
myHashtable.Keys.CopyTo(myKeys, 0);
for (int counter = 0; counter < myKeys.Length; counter++)
{
Console.WriteLine("myKeys[" + counter + "] = " +
myKeys[counter]);
}
// copy the values from myHashtable into an array using
// the CopyTo() method and then display the array contents
Console.WriteLine("Copying values to myValues array");
string[] myValues = new string[count];
myHashtable.Values.CopyTo(myValues, 0);
for (int counter = 0; counter < myValues.Length; counter++)
{
Console.WriteLine("myValues[" + counter + "] = " +
myValues[counter]);
}
}
}
Related examples in the same category