implements the IComparable interface
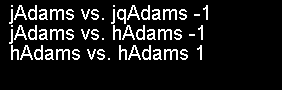
using System;
public class NewOrderedName : IComparable {
private String firstName;
private String lastName;
public NewOrderedName(String f, String l) {
firstName = f;
lastName = l;
}
public int CompareTo(Object o) {
NewOrderedName name = (NewOrderedName)o;
int lastResult = lastName.CompareTo(name.lastName);
if (lastResult != 0)
return lastResult;
else {
int firstResult = firstName.CompareTo(name.firstName);
if (firstResult != 0)
return firstResult;
else
return 1;
}
}
public static void Main() {
NewOrderedName jAdams = new NewOrderedName("J", "A");
NewOrderedName jqAdams = new NewOrderedName("A", "Q");
NewOrderedName hAdams = new NewOrderedName("H", "S");
Console.WriteLine ("jAdams vs. jqAdams {0}", jAdams.CompareTo(jqAdams));
Console.WriteLine ("jAdams vs. hAdams {0}", jAdams.CompareTo(hAdams));
Console.WriteLine ("hAdams vs. hAdams {0}", hAdams.CompareTo(hAdams));
}
}
Related examples in the same category