the use of a three-dimensional rectangular array
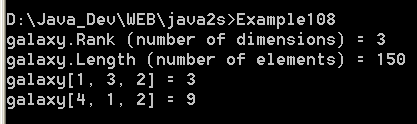
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example10_8.cs illustrates the use of
a three-dimensional rectangular array
*/
using System;
public class Example10_8
{
public static void Main()
{
// create the galaxy array
int[,,] galaxy = new int [10, 5, 3];
// set two galaxy array elements to the star's brightness
galaxy[1, 3, 2] = 3;
galaxy[4, 1, 2] = 9;
// display the Rank and Length properties of the galaxy array
Console.WriteLine("galaxy.Rank (number of dimensions) = " + galaxy.Rank);
Console.WriteLine("galaxy.Length (number of elements) = " + galaxy.Length);
// display the galaxy array elements, but only display elements that
// have actually been set to a value (or "contain stars")
for (int x = 0; x < galaxy.GetLength(0); x++)
{
for (int y = 0; y < galaxy.GetLength(1); y++)
{
for (int z = 0; z < galaxy.GetLength(2); z++)
{
if (galaxy[x, y, z] != 0)
{
Console.WriteLine("galaxy[" + x + ", " + y + ", " + z +"] = " +
galaxy[x, y, z]);
}
}
}
}
}
}
Related examples in the same category