Demonstrate string arrays
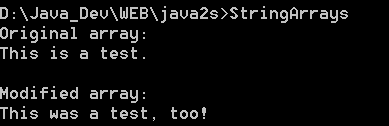
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate string arrays.
using System;
public class StringArrays {
public static void Main() {
string[] str = { "This", "is", "a", "test." };
Console.WriteLine("Original array: ");
for(int i=0; i < str.Length; i++)
Console.Write(str[i] + " ");
Console.WriteLine("\n");
// change a string
str[1] = "was";
str[3] = "test, too!";
Console.WriteLine("Modified array: ");
for(int i=0; i < str.Length; i++)
Console.Write(str[i] + " ");
}
}
Related examples in the same category