Illustrates boxing and unboxing
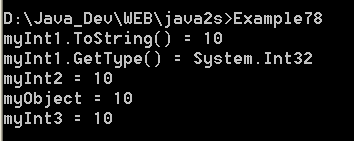
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_8.cs illustrates boxing and unboxing
*/
using System;
public class Example7_8
{
public static void Main()
{
// implicit boxing of an int
int myInt1 = 10;
Console.WriteLine("myInt1.ToString() = " + myInt1.ToString());
Console.WriteLine("myInt1.GetType() = " + myInt1.GetType());
// explicit boxing of an int to an object
int myInt2 = 10;
object myObject = myInt2; // myInt2 is boxed
Console.WriteLine("myInt2 = " + myInt2);
Console.WriteLine("myObject = " + myObject);
// explicit unboxing of an object to an int
int myInt3 = (int) myObject; // myObject is unboxed
Console.WriteLine("myInt3 = " + myInt3);
}
}
Related examples in the same category