Is a char in a range: Case Insensitive
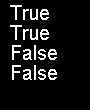
using System;
using System.Data;
using System.Text.RegularExpressions;
using System.Text;
class Class1{
static void Main(string[] args){
Console.WriteLine(IsInRangeCaseInsensitive('c', 'a', 'G'));
Console.WriteLine(IsInRangeCaseInsensitive('c', 'a', 'c'));
Console.WriteLine(IsInRangeCaseInsensitive('c', 'g', 'g'));
Console.WriteLine(IsInRangeCaseInsensitive((char)32, 'a', 'b'));
}
public static bool IsInRangeCaseInsensitive(char testChar, char startOfRange, char endOfRange)
{
testChar = char.ToUpper(testChar);
startOfRange = char.ToUpper(startOfRange);
endOfRange = char.ToUpper(endOfRange);
if (testChar >= startOfRange && testChar <= endOfRange)
{
// testChar is within the range
return (true);
}
else
{
// testChar is NOT within the range
return (false);
}
}
}
Related examples in the same category
1. | Get char type: control, digit, letter, number, punctuation, surrogate, symbol and white space | | 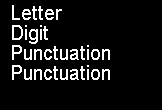 |
2. | Determining If A Character Is Within A Specified Range | | 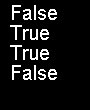 |
3. | Is a char in a range Exclusively | |  |
4. | Using Char | | |
5. | Escape Characters | | |
6. | A stack class for characters | | 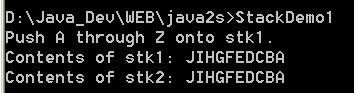 |
7. | Encode or decode a message | | |
8. | Demonstrate several Char methods | | 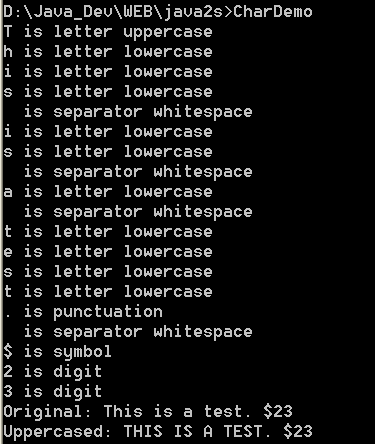 |
9. | Demonstrate the ICharQ interface: A character queue interface | | 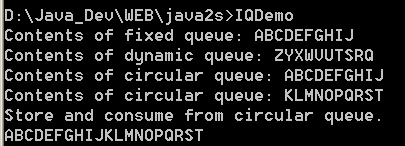 |
10. | A set class for characters | | 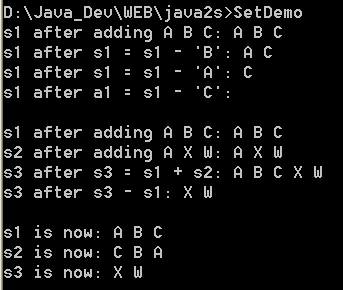 |
11. | A queue class for characters | | 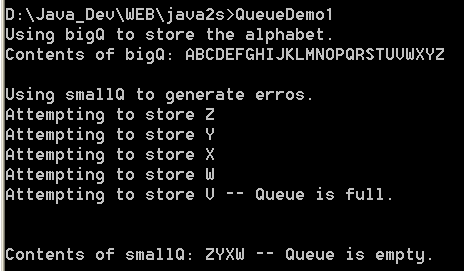 |
12. | IsDigit, IsLetter, IsWhiteSpace, IsLetterOrDigit, IsPunctuation | | |
13. | Char: Get Unicode Category | | |
14. | Char.IsLowSurrogate(), IsHighSurrogate(), IsSurrogatePair() method | | |
15. | demonstrates IsSymbol. | | |
16. | Buffer for characters | | |
17. | Test an input character if it is contained in a character list. | | |
18. | Is vowel char | | |
19. | Filter letter and digit | | |
20. | First Char Upper | | |