Obtaining the Most Significant or Least Significant Bits of a Number
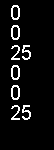
using System;
using System.Data;
class Class1{
static void Main(string[] args){
int number = 25;
short num = 25;
Console.WriteLine(GetMSB(number));
Console.WriteLine(GetConvertMSB(number));
Console.WriteLine(GetLSB(number));
Console.WriteLine(GetConvertLSB(number));
Console.WriteLine(GetMSB(num));
Console.WriteLine(GetLSB(num));
}
public static int GetMSB(int value)
{
return (int)(value & 0xFFFF0000);
}
public static int GetConvertMSB(int value)
{
return (value & Convert.ToInt32("11111111111111110000000000000000", 2));
}
public static int GetLSB(int intValue)
{
return (intValue & 0x0000FFFF);
}
public static int GetConvertLSB(int intValue)
{
return (intValue & Convert.ToInt32("11111111111111110000000000000000", 2));
}
public static int GetMSB(short intValue)
{
return (intValue & 0xFF00);
}
public static int GetLSB(short intValue)
{
return (intValue & 0x00FF);
}
}
Related examples in the same category