Size of int, double, char and bool
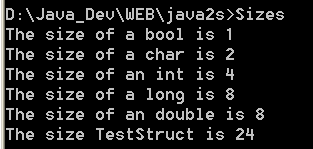
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
//
// Sizes.cs -- returns the sixes of C# data types.
// Compile with the following command line:
// csc /unsafe sizes.cs
//
namespace nsSizes
{
using System;
struct TestStruct
{
int x;
double y;
char ch;
}
public class Sizes
{
static public unsafe void Main ()
{
Console.WriteLine ("The size of a bool is " + sizeof (bool));
Console.WriteLine ("The size of a char is " + sizeof (char));
Console.WriteLine ("The size of an int is " + sizeof (int));
Console.WriteLine ("The size of a long is " + sizeof (long));
Console.WriteLine ("The size of an double is " + sizeof (double));
Console.WriteLine ("The size TestStruct is " + sizeof (TestStruct));
}
}
}
Related examples in the same category