use the Equals() method and equality operator to check if two strings are equal
using System;
class MainClass {
public static void Main() {
string myString = String.Concat("Friends, ", "Romans");
string myString2 = String.Concat("Friends");
bool boolResult;
boolResult = String.Equals("bbc", "bbc");
Console.WriteLine("String.Equals(\"bbc\", \"bbc\") is " + boolResult);
boolResult = myString.Equals(myString2);
Console.WriteLine("myString.Equals(myString2) is " + boolResult);
boolResult = myString == myString2;
Console.WriteLine("myString == myString2 is " + boolResult);
}
}
Related examples in the same category
1. | Test for equality between stings. | | |
2. | use the Compare() method to compare strings | | |
3. | Comparing a String to the Beginning or End of a Second String | | 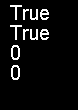 |
4. | Compare strings | | 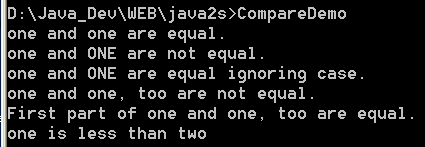 |
5. | String copy and equal | | 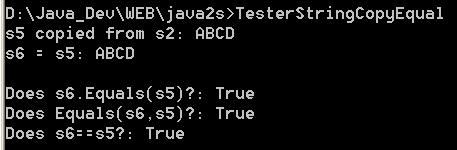 |
6. | Use String.Compare to check if a URI is a file URI | | |
7. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), ignore case | | |
8. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), Honor case | | |
9. | Check if a String contain another string by ignoring case | | |
10. | Compare two strings with StringComparison.CurrentCultureIgnoreCase | | |
11. | Checks if the string starts with special character. This does not include any special character from AllowedSpecialCharacters | | |