Converts the file time to an equivalent local time.
using System;
using System.IO;
using System.Runtime.InteropServices;
public struct FileTime
{
public uint dwLowDateTime;
public uint dwHighDateTime;
public static implicit operator long(FileTime fileTime)
{
long returnedLong;
byte[] highBytes = BitConverter.GetBytes(fileTime.dwHighDateTime);
Array.Resize(ref highBytes, 8);
returnedLong = BitConverter.ToInt64(highBytes, 0);
returnedLong = returnedLong << 32;
returnedLong = returnedLong | fileTime.dwLowDateTime;
return returnedLong;
}
}
public class FileTimes
{
private const int OPEN_EXISTING = 3;
private const int INVALID_HANDLE_VALUE = -1;
[DllImport("Kernel32.dll", CharSet = CharSet.Unicode)]
private static extern int CreateFile(string lpFileName,
int dwDesiredAccess,
int dwShareMode,
int lpSecurityAttributes,
int dwCreationDisposition,
int dwFlagsAndAttributes,
int hTemplateFile);
[DllImport("Kernel32.dll")]
private static extern bool GetFileTime(int hFile,
out FileTime lpCreationTime,
out FileTime lpLastAccessTime,
out FileTime lpLastWriteTime);
[DllImport("Kernel32.dll")]
private static extern bool CloseHandle(int hFile);
public static void Main()
{
string winDir = Environment.SystemDirectory;
if (! (winDir.EndsWith(Path.DirectorySeparatorChar.ToString())))
winDir += Path.DirectorySeparatorChar;
winDir += "write.exe";
int hFile = CreateFile(winDir, 0, 0, 0, OPEN_EXISTING, 0, 0);
if (hFile == INVALID_HANDLE_VALUE)
{
Console.WriteLine("Unable to access {0}.", winDir);
}
else
{
FileTime creationTime, accessTime, writeTime;
if (GetFileTime(hFile, out creationTime, out accessTime, out writeTime))
{
CloseHandle(hFile);
long fileCreationTime = (long) creationTime;
long fileAccessTime = accessTime;
long fileWriteTime = (long) writeTime;
Console.WriteLine(DateTimeOffset.FromFileTime(fileCreationTime).ToString());
Console.WriteLine(DateTimeOffset.FromFileTime(fileAccessTime).ToString());
Console.WriteLine(DateTimeOffset.FromFileTime(fileWriteTime).ToString());
}
}
FileInfo fileInfo = new FileInfo(winDir);
DateTimeOffset infoCreationTime, infoAccessTime, infoWriteTime;
long ftCreationTime, ftAccessTime, ftWriteTime;
infoCreationTime = fileInfo.CreationTime;
infoAccessTime = fileInfo.LastAccessTime;
infoWriteTime = fileInfo.LastWriteTime;
ftCreationTime = infoCreationTime.ToFileTime();
ftAccessTime = infoAccessTime.ToFileTime();
ftWriteTime = infoWriteTime.ToFileTime();
Console.WriteLine(DateTimeOffset.FromFileTime(ftCreationTime).ToString());
Console.WriteLine(DateTimeOffset.FromFileTime(ftAccessTime).ToString());
Console.WriteLine(DateTimeOffset.FromFileTime(ftWriteTime).ToString());
}
}
Related examples in the same category
1. | Create DateTimeOffset using the specified DateTime value. | | |
2. | Create DateTimeOffset using the specified DateTime value and offset. | | |
3. | Create DateTimeOffset using the specified year, month, day, hour, minute, second, millisecond, and offset of a specified calendar. | | |
4. | Create DateTimeOffset using the specified year, month, day, hour, minute, second, millisecond, and offset. | | |
5. | Create DateTimeOffset using the specified year, month, day, hour, minute, second, and offset. | | |
6. | Create DateTimeOffset using the specified number of ticks and offset. | | |
7. | Gets hour from DateTimeOffset | | 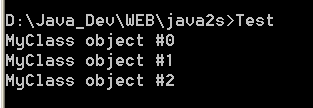 |
8. | Gets second from DateTimeOffset | | 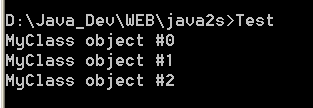 |
9. | Gets second from DateTimeOffset: s (second) | | 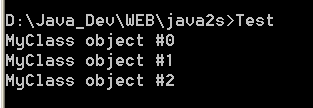 |
10. | Gets second from DateTimeOffset: ss | | 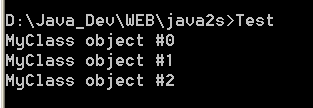 |
11. | Gets year from DateTimeOffset | | |
12. | Converts DateTimeOffset to the date and time specified by an offset value. | | |
13. | Converts DateTimeOffset to string with ToString() method | | |
14. | Converts DateTimeOffset to a DateTimeOffset value representing the Coordinated Universal Time (UTC). | | |
15. | Compare two DateTimeOffset values for equality | | 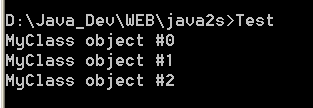 |
16. | Compare two DateTimeOffset values | | 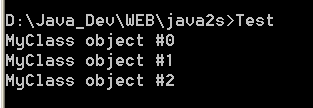 |
17. | Compare one DateTimeOffset with another object | | 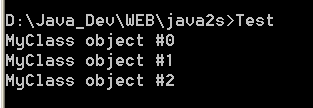 |
18. | Compare two DateTimeOffset values for time and offset | | 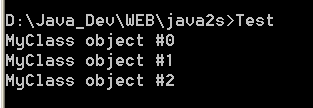 |
19. | Show possible time zone for a DateTimeOffset | | |
20. | Create DateTimeOffset from DateTime with TimeSpan | | |
21. | Choosing Between DateTime, DateTimeOffset, and TimeZoneInfo | | |
22. | Find difference between Date.Now and Date.UtcNow | | |
23. | Find difference between Now and UtcNow using DateTimeOffset | | |
24. | Compares two DateTimeOffset objects and indicates the order. | | |
25. | Compares current DateTimeOffset to a specified DateTimeOffset object and indicates the order. | | |
26. | Gets a DateTime value from DateTimeOffset | | 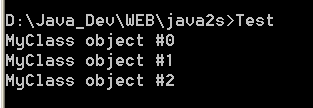 |
27. | Gets the day of the month from DateTimeOffset object. | | 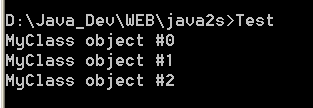 |
28. | Gets the day of the week from DateTimeOffset | | 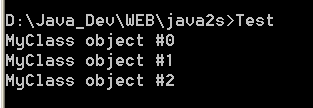 |
29. | Gets a DateTime value that represents the local date and time from DateTimeOffset | | 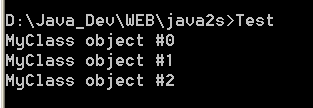 |
30. | Transition to DST in local time zone occurs on 3/11/2007 at 2:00 AM | | 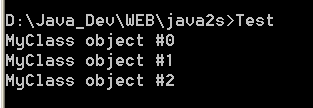 |
31. | Is valid local time | | 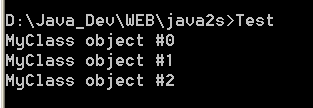 |
32. | Is it an ambiguous time | | 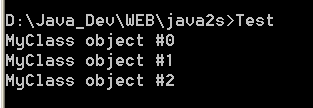 |
33. | Gets millisecond from DateTimeOffset | | 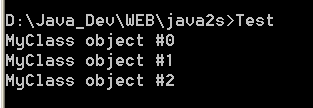 |
34. | Gets minute from DateTimeOffset | | 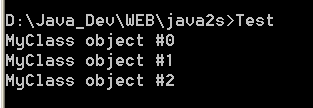 |
35. | Gets the month from DateTimeOffset | | 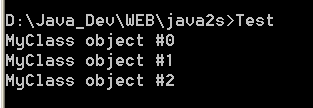 |
36. | Gets current date and time with the local time's offset from Coordinated Universal Time (UTC). | | 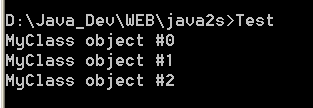 |
37. | Gets the time's offset from Coordinated Universal Time (UTC). | | 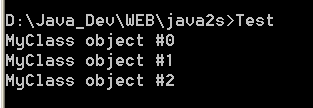 |
38. | Assume time is local during the parse | | 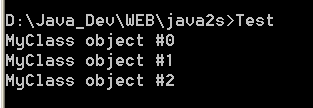 |
39. | Assume time is UTC | | 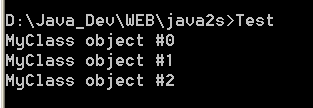 |
40. | Parse and convert to UTC | | 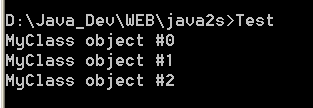 |
41. | Gets the number of ticks from DateTimeOffset | | 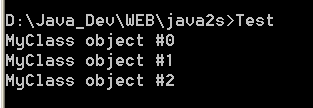 |
42. | Gets the time of day from DateTimeOffset | | 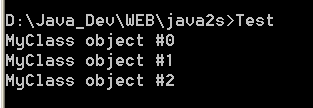 |
43. | A summer UTC time vs a winter time | | 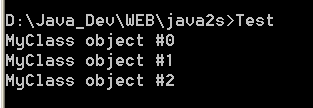 |
44. | Get current time in UTC | | |