DateTimeFormatInfo.CurrentInfo.TimeSeparator
using System;
using System.Globalization;
using System.Text.RegularExpressions;
using System.Threading;
public class Example
{
public static void Main()
{
Console.WriteLine("Using the Persian Calendar:");
PersianCalendar persian = new PersianCalendar();
DateTime date1 = new DateTime(2010, 5, 22, 11, 31, 11, 500, persian);
Console.WriteLine(date1.ToString("M/dd/yyyy h:mm:ss.fff tt"));
Console.WriteLine("{0}/{1}/{2} {3}{7}{4:D2}{7}{5:D2}.{6:G3}\n",
persian.GetMonth(date1),
persian.GetDayOfMonth(date1),
persian.GetYear(date1),
persian.GetHour(date1),
persian.GetMinute(date1),
persian.GetSecond(date1),
persian.GetMilliseconds(date1),
DateTimeFormatInfo.CurrentInfo.TimeSeparator);
}
}
// Using the Persian Calendar:
// 8/13/2631 11:31:11.500 AM
// 5/22/2010 11:31:11.500
Related examples in the same category
1. | Current date and time | | |
2. | What day of the month is this? | | |
3. | Do some leap year checks | | |
4. | Look at the min and max date/time values | | |
5. | Output DateTime object | | |
6. | Constructors of DateTime | | |
7. | comparisons between DateTime objects | | |
8. | Parse and ParseExact | | |
9. | DateTime Now and its calculation | | |
10. | new DateTime(1900, 2, 29) | | |
11. | new DateTime(1900, 2, 29, new JulianCalendar()) | | |
12. | Specify Kind DateTime | | |
13. | Offset of DateTime | | |
14. | DateTime and TimeSpan Instances | | |
15. | use the Now and UtcNow properties to get the currrent date and time | | |
16. | display the Date, Day, DayOfWeek, DayOfYear,Ticks, and TimeOfDayProperties of myDateTime | | |
17. | use the Compare() method to compare DateTime instances | | |
18. | use the overloaded less than operator (<) to compare two DateTime instances | | |
19. | use the Equals() method to compare DateTime instances | | |
20. | use the DaysInMonth() method to retrieve the number of days in a particular month and year | | |
21. | use the IsLeapYear() method to determine if a particular year is a leap year | | |
22. | use the Parse() method to convert strings to DateTime instances | | |
23. | use the Subtract() method to subtract a TimeSpan from a DateTime | | |
24. | use the overloaded subtraction operator (-) to subtract a TimeSpan from a DateTime | | |
25. | Illustrates the use of DateTime and TimeSpan instances | | 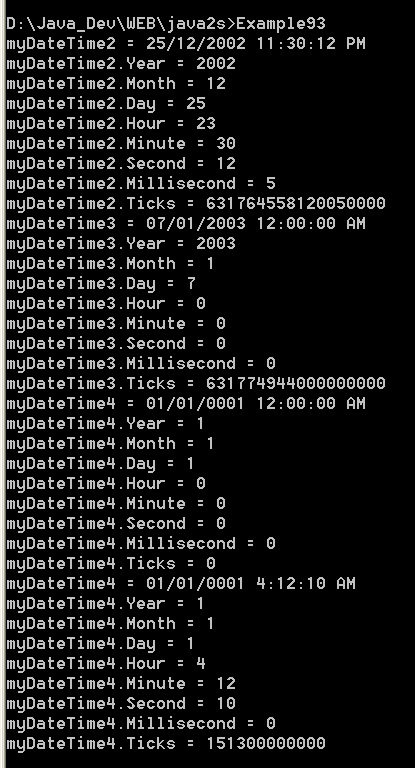 |
26. | Displays the words 'Hello World!' on the screen, along with the current date and time | |  |
27. | illustrates the use of TimeSpan properties and methods | | 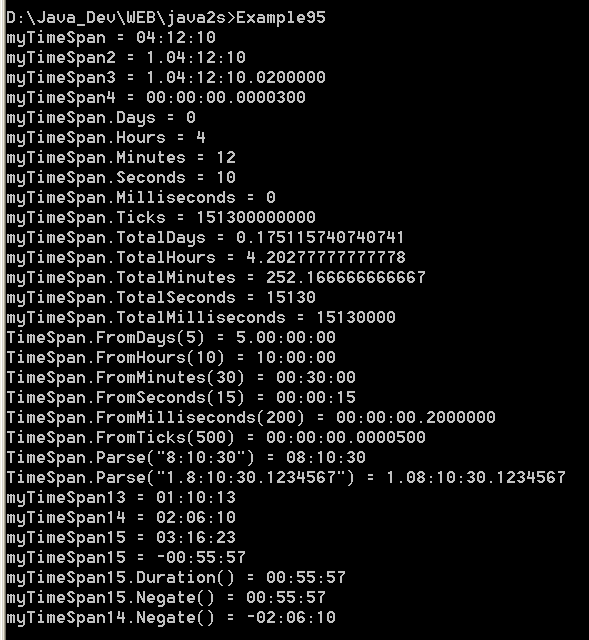 |
28. | A simple clock | | 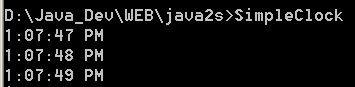 |
29. | Estimates pi by throwing points into a square. Use to
compare execution times | |  |
30. | Change current culture and back | | |
31. | How values you passed into contructor mapped to its Properties | | |
32. | Gets the day of the week | | |
33. | Returns the number of days in the specified month and year. | | |
34. | DateTime and == | | |
35. | Create a DateTime structure with local time | | |
36. | Compare DateTime instance with Equal methods | | |
37. | Format a DateTime with M/dd/yyyy h:mm:ss.fff tt | | |
38. | Check the Kind of DateTime | | |
39. | Initializes a DateTime structure to a specified number of ticks. | | |
40. | DateTime.Now, DateTime.UtcNow, DateTime.Today | | |
41. | Parse a date time value from a string with Invariant Culture | | |
42. | Default value of DateTime is DateTime.MinValue | | |
43. | Default ToString output | | |
44. | Convert DateTime to string with fr-FR culture | | |
45. | ToString single letter format: F | | |
46. | DateTime ToString single letter format with fr-FR culture | | |
47. | DateTime Compare | | |
48. | DateTime CompareTo | | |
49. | Gets the date component of this instance. | | |
50. | Time String | | |
51. | Create day and year for a certain month | | |
52. | Returns the number of seconds since January 1, 1970 | | |