Demonstrates principal and identity objects
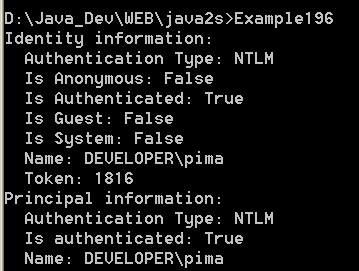
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example19_6.cs demonstrates principal & identity objects
*/
using System;
using System.Security.Principal;
public class Example19_6
{
public static void Main()
{
// get the current identity
WindowsIdentity wi = WindowsIdentity.GetCurrent();
Console.WriteLine("Identity information:");
Console.WriteLine(" Authentication Type: {0}",wi.AuthenticationType);
Console.WriteLine(" Is Anonymous: {0}", wi.IsAnonymous);
Console.WriteLine(" Is Authenticated: {0}", wi.IsAuthenticated);
Console.WriteLine(" Is Guest: {0}", wi.IsGuest);
Console.WriteLine(" Is System: {0}", wi.IsSystem);
Console.WriteLine(" Name: {0}", wi.Name);
Console.WriteLine(" Token: {0}", wi.Token);
// get the associated principal
WindowsPrincipal prin = new WindowsPrincipal(wi);
Console.WriteLine("Principal information:");
Console.WriteLine(" Authentication Type: {0}", prin.Identity.AuthenticationType);
Console.WriteLine(" Is authenticated: {0}", prin.Identity.IsAuthenticated);
Console.WriteLine(" Name: {0}", prin.Identity.Name);
}
}
Related examples in the same category