illustrates some of the System.Object class methods
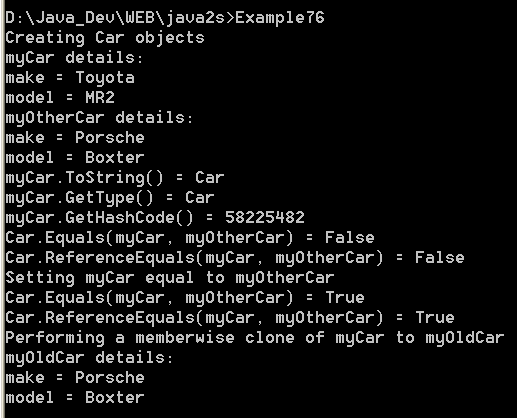
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_6.cs illustrates some of the System.Object
class methods
*/
using System;
// declare the Car class
class Car
{
// declare the fields
public string make;
public string model;
// define a constructor
public Car(string make, string model)
{
this.make = make;
this.model = model;
}
// define the Display() method
public void Display()
{
Console.WriteLine("make = " + make);
Console.WriteLine("model = " + model);
}
// define the Copy() method
public static Car Copy(Car car)
{
// perform memberwise clone
return (Car) car.MemberwiseClone();
}
}
public class Example7_6
{
public static void Main()
{
// create Car objects
Console.WriteLine("Creating Car objects");
Car myCar = new Car("Toyota", "MR2");
Car myOtherCar = new Car("Porsche", "Boxter");
Console.WriteLine("myCar details:");
myCar.Display();
Console.WriteLine("myOtherCar details:");
myOtherCar.Display();
// call some of the methods inherited from the System.Object class
Console.WriteLine("myCar.ToString() = " + myCar.ToString());
Console.WriteLine("myCar.GetType() = " + myCar.GetType());
Console.WriteLine("myCar.GetHashCode() = " + myCar.GetHashCode());
Console.WriteLine("Car.Equals(myCar, myOtherCar) = " +
Car.Equals(myCar, myOtherCar));
Console.WriteLine("Car.ReferenceEquals(myCar, myOtherCar) = " +
Car.ReferenceEquals(myCar, myOtherCar));
// set the myCar object reference equal to myOtherCar
Console.WriteLine("Setting myCar equal to myOtherCar");
myCar = myOtherCar;
// check for equality
Console.WriteLine("Car.Equals(myCar, myOtherCar) = " +
Car.Equals(myCar, myOtherCar));
Console.WriteLine("Car.ReferenceEquals(myCar, myOtherCar) = " +
Car.ReferenceEquals(myCar, myOtherCar));
// perform a memberwise clone of myCar using the Car.Copy() method
Console.WriteLine("Performing a memberwise clone of myCar to myOldCar");
Car myOldCar = Car.Copy(myCar);
Console.WriteLine("myOldCar details:");
myOldCar.Display();
}
}
Related examples in the same category