Key Press
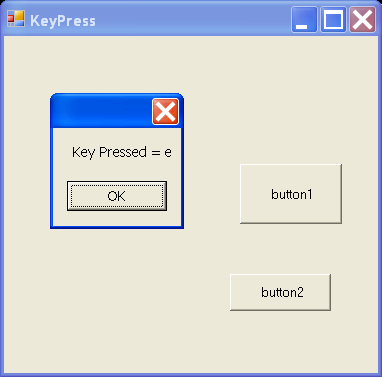
/*
GDI+ Programming in C# and VB .NET
by Nick Symmonds
Publisher: Apress
ISBN: 159059035X
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace KeyPress
{
/// <summary>
/// Summary description for KeyPress.
/// </summary>
public class KeyPress : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public KeyPress()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(184, 104);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(80, 48);
this.button1.TabIndex = 0;
this.button1.Text = "button1";
//
// button2
//
this.button2.Location = new System.Drawing.Point(176, 192);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(80, 32);
this.button2.TabIndex = 1;
this.button2.Text = "button2";
//
// KeyPress
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button2,
this.button1});
this.Name = "KeyPress";
this.Text = "KeyPress";
this.Load += new System.EventHandler(this.KeyPress_Load);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new KeyPress());
}
private void KeyPress_Load(object sender, System.EventArgs e)
{
this.KeyPreview=true;
}
protected override void OnKeyPress(KeyPressEventArgs e)
{
MessageBox.Show("Key Pressed = " + e.KeyChar.ToString());
}
}
}
Related examples in the same category