Create Directory
/*
* FCKeditor - The text editor for Internet - http://www.fckeditor.net
* Copyright (C) 2003-2007 Frederico Caldeira Knabben
*
* == BEGIN LICENSE ==
*
* Licensed under the terms of any of the following licenses at your
* choice:
*
* - GNU General Public License Version 2 or later (the "GPL")
* http://www.gnu.org/licenses/gpl.html
*
* - GNU Lesser General Public License Version 2.1 or later (the "LGPL")
* http://www.gnu.org/licenses/lgpl.html
*
* - Mozilla Public License Version 1.1 or later (the "MPL")
* http://www.mozilla.org/MPL/MPL-1.1.html
*
* == END LICENSE ==
*
* Useful tools.
*/
using System ;
using System.Runtime.InteropServices ;
using System.IO ;
using System.Collections ;
namespace FredCK.FCKeditorV2
{
internal sealed class Util
{
// The "_mkdir" function is used by the "CreateDirectory" method.
[DllImport("msvcrt.dll", SetLastError=true)]
private static extern int _mkdir(string path) ;
private Util()
{}
/// <summary>
/// This method should provide safe substitude for Directory.CreateDirectory().
/// </summary>
/// <param name="path">The directory path to be created.</param>
/// <returns>A <see cref="System.IO.DirectoryInfo"/> object for the created directory.</returns>
/// <remarks>
/// <para>
/// This method creates all the directory structure if needed.
/// </para>
/// <para>
/// The System.IO.Directory.CreateDirectory() method has a bug that gives an
/// error when trying to create a directory AND the user has no rights defined
/// in one of its parent directories. The CreateDirectory() should be a good
/// replacement to solve this problem.
/// </para>
/// </remarks>
public static DirectoryInfo CreateDirectory( string path )
{
// Create the directory info object for that dir (normalized to its absolute representation).
DirectoryInfo oDir = new DirectoryInfo( Path.GetFullPath( path ) ) ;
try
{
// Try to create the directory by using standard .Net features. (#415)
if ( !oDir.Exists )
oDir.Create();
return oDir;
}
catch
{
CreateDirectoryUsingDll( oDir );
return new DirectoryInfo( path );
}
}
private static void CreateDirectoryUsingDll( DirectoryInfo dir )
{
// On some occasion, the DirectoryInfo.Create() function will
// throw an error due to a bug in the .Net Framework design. For
// example, it may happen that the user has no permissions to
// list entries in a lower level in the directory path, AND the
// Create() call will simply fail.
// To workaround it, we use mkdir directly.
ArrayList oDirsToCreate = new ArrayList();
// Check the entire path structure to find directories that must be created.
while ( dir != null && !dir.Exists )
{
oDirsToCreate.Add( dir.FullName );
dir = dir.Parent;
}
// "dir == null" means that the check arrives in the root AND it doesn't exist too.
if ( dir == null )
throw ( new System.IO.DirectoryNotFoundException( "Directory \"" + oDirsToCreate[ oDirsToCreate.Count - 1 ] + "\" not found." ) );
// Create all directories that must be created (from bottom to top).
for ( int i = oDirsToCreate.Count - 1 ; i >= 0 ; i-- )
{
string sPath = (string)oDirsToCreate[ i ];
int iReturn = _mkdir( sPath );
if ( iReturn != 0 )
throw new ApplicationException( "Error calling [msvcrt.dll]:_wmkdir(" + sPath + "), error code: " + iReturn );
}
}
public static bool ArrayContains( Array array, object value, System.Collections.IComparer comparer )
{
foreach ( object item in array )
{
if ( comparer.Compare( item, value ) == 0 )
return true;
}
return false;
}
}
}
Related examples in the same category
1. | Find Files That Match a Wildcard Expression | | |
2. | Get Files from a directory | | |
3. | Get Directory properties from DirectionInfo class | | |
4. | Get directory name and file information in that directory | | |
5. | Directory Counter | | |
6. | File Search | | |
7. | Get Name, Parent, Exists properties from DirectoryInfo class | | |
8. | Get Creation Time | | |
9. | Get Last Write Time | | |
10. | Get Last Access Time | | |
11. | Calculate Directory Size | | |
12. | Copy Directory | | |
13. | Check the Existance of a Directory | | |
14. | Get Current Directory | | |
15. | Set Current Directory | | |
16. | Traversing Directories | | 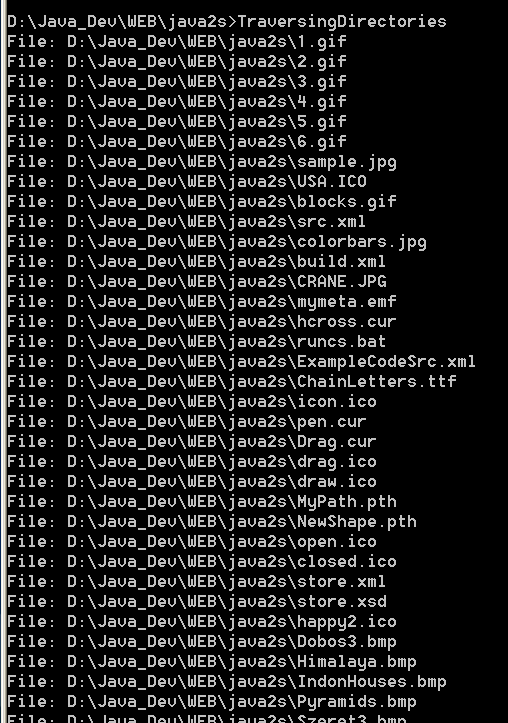 |
17. | Directory Object | | |
18. | illustrates recursive Directory use | | 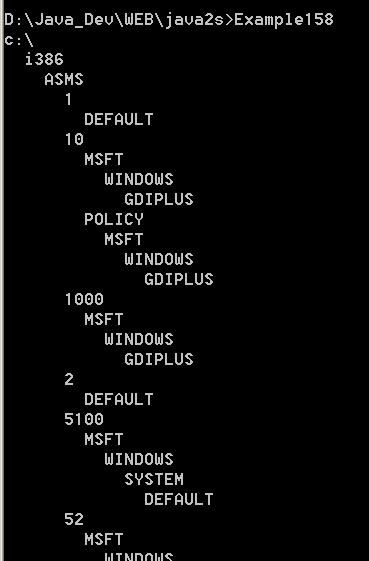 |
19. | illustrates the Directory class | | 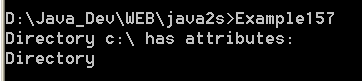 |
20. | illustrates the Directory class 2 | | 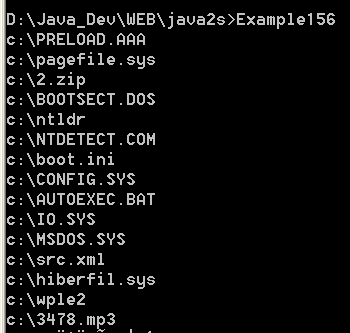 |
21. | Uses the DirectoryInfo class to recursively show subdirectories | | 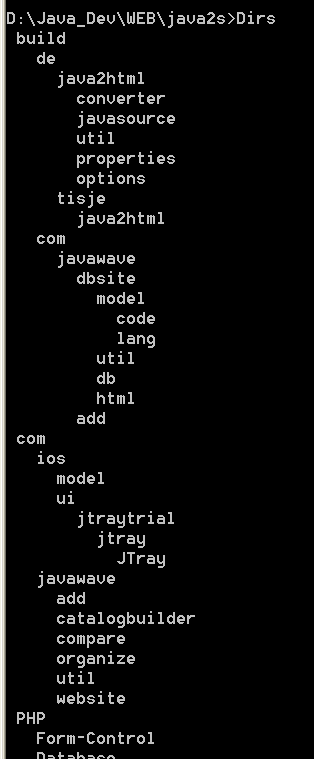 |
22. | Changes the current working directory and then lists the files in the directory | | 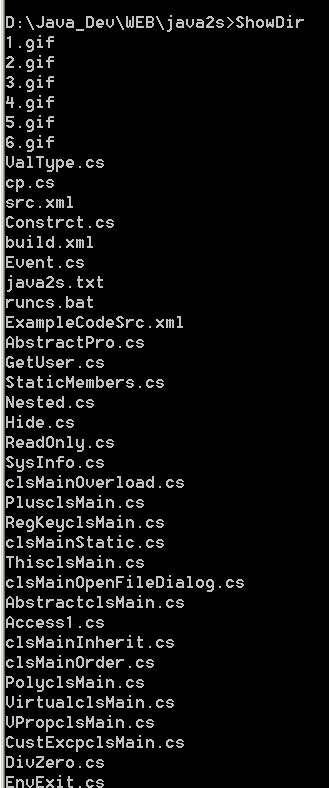 |
23. | Directory Tree Host | | 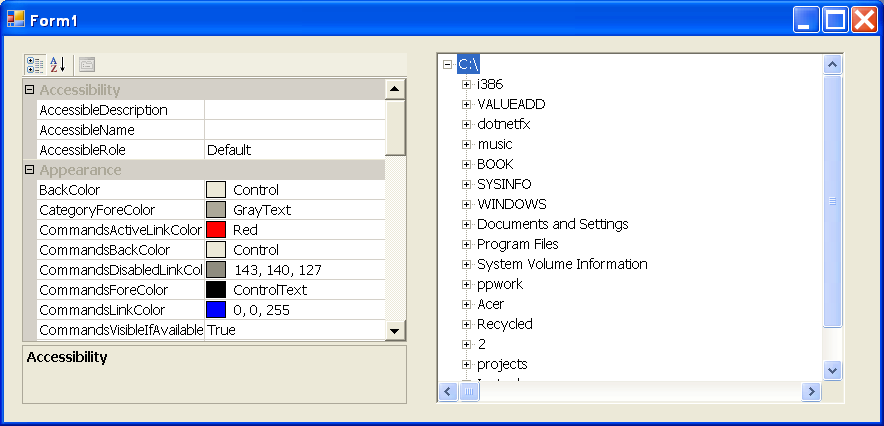 |
24. | Gets the size of all files within a directory | | |
25. | Determine if the directory is empty, ie. no files and no sub-directories | | |
26. | Get an array of files info from a directory. | | |
27. | Removes a directory as best as it can. Errors are ignored. | | |
28. | Directory Class xxposes static methods for creating, moving, and enumerating through directories and subdirectories. | | |
29. | Calculates the size of a directory and its subdirectories, if any, and displays the total size in bytes. | | |
30. | Gets the current working directory of the application. | | |
31. | Directory Class | | |
32. | Calculate the size of a directory and its subdirectories, if any, and displays the total size in bytes. | | |
33. | Creates all directories and subdirectories in the specified path. | | |
34. | Create new nested directories | | |
35. | Deletes an empty directory from a specified path. | | |
36. | Deletes the directory any subdirectories and files in the directory. | | |
37. | Returns an enumerable collection of directory names in a specified path. | | |
38. | Returns an enumerable collection of directory names that match a search pattern in a specified path. | | |
39. | Get collection of directory names that match a search pattern, and optionally searches subdirectories. | | |
40. | Returns an enumerable collection of file names in a specified path. | | |
41. | Returns an enumerable collection of file names that match a search pattern in a specified path. | | |
42. | Get a collection of file names that match a search pattern, and optionally searches subdirectories. | | |
43. | Determines whether the given path refers to an existing directory on disk. | | |
44. | Gets a DirectorySecurity object that encapsulates the access control list (ACL) entries | | |
45. | Gets the creation date and time of a directory. | | |
46. | Gets the creation date and time, in Coordinated Universal Time (UTC) format, of a directory. | | |
47. | Gets the names of subdirectories in the specified directory. | | |
48. | Gets an array of directories matching the specified search pattern from the current directory. | | |
49. | Returns the volume information, root information, or both for the specified path. | | |
50. | Returns the names of files that match the specified search pattern | | |
51. | Returns the names of all files and subdirectories in the specified directory. | | |
52. | Returns the date and time the specified file or directory was last accessed. | | |
53. | Returns the date and time the specified file or directory was last written to. | | |
54. | Sets the date and time the specified file or directory was last accessed. | | |
55. | Sets the date and time a directory was last written to. | | |
56. | Instance methods for creating, moving, and enumerating through directories and subdirectories. | | |
57. | Copy directories with DirectoryInfo | | |
58. | DriveInfo Class Provides access to information on a drive. | | |
59. | Read and Write to a Newly Created Data File | | |
60. | Read Text from a File | | |
61. | File.OpenText returns a StreamReader | | |
62. | Write Text to a File | | |
63. | Copy,delete file and directory | | |
64. | Find all files in a directory, and all files within every nested directory. | | |
65. | Find all files in a directory, and all files within every nested directory. (2) | | |
66. | Current Dir | | |
67. | Directory Walker | | |
68. | Class, which describes folder with its subfolders. | | |