Demonstrate random access
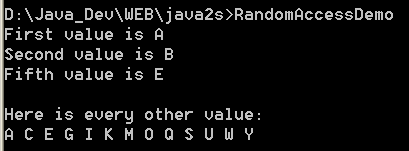
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate random access.
using System;
using System.IO;
public class RandomAccessDemo {
public static void Main() {
FileStream f;
char ch;
try {
f = new FileStream("random.dat", FileMode.Create);
}
catch(IOException exc) {
Console.WriteLine(exc.Message);
return ;
}
// Write the alphabet.
for(int i=0; i < 26; i++) {
try {
f.WriteByte((byte)('A'+i));
}
catch(IOException exc) {
Console.WriteLine(exc.Message);
return ;
}
}
try {
// Now, read back specific values
f.Seek(0, SeekOrigin.Begin); // seek to first byte
ch = (char) f.ReadByte();
Console.WriteLine("First value is " + ch);
f.Seek(1, SeekOrigin.Begin); // seek to second byte
ch = (char) f.ReadByte();
Console.WriteLine("Second value is " + ch);
f.Seek(4, SeekOrigin.Begin); // seek to 5th byte
ch = (char) f.ReadByte();
Console.WriteLine("Fifth value is " + ch);
Console.WriteLine();
// Now, read every other value.
Console.WriteLine("Here is every other value: ");
for(int i=0; i < 26; i += 2) {
f.Seek(i, SeekOrigin.Begin); // seek to ith double
ch = (char) f.ReadByte();
Console.Write(ch + " ");
}
}
catch(IOException exc) {
Console.WriteLine(exc.Message);
}
Console.WriteLine();
f.Close();
}
}
Related examples in the same category