Text Reader
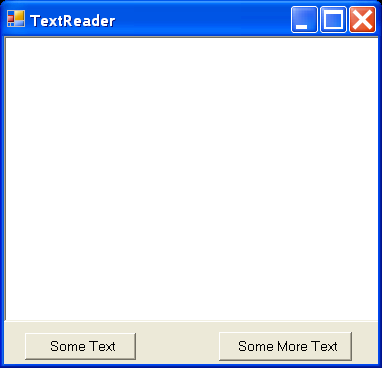
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;
using System.Text;
namespace Wrox.WindowsGUIProgramming.Chapter10
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class TextReader : System.Windows.Forms.Form
{
private System.Windows.Forms.RichTextBox rtbText;
private System.Windows.Forms.Button butSomeText;
private System.Windows.Forms.Button butSomeMoreText;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public TextReader()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.rtbText = new System.Windows.Forms.RichTextBox();
this.butSomeText = new System.Windows.Forms.Button();
this.butSomeMoreText = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// rtbText
//
this.rtbText.Name = "rtbText";
this.rtbText.Size = new System.Drawing.Size(296, 232);
this.rtbText.TabIndex = 0;
this.rtbText.Text = "";
//
// butSomeText
//
this.butSomeText.Location = new System.Drawing.Point(16, 240);
this.butSomeText.Name = "butSomeText";
this.butSomeText.Size = new System.Drawing.Size(88, 23);
this.butSomeText.TabIndex = 1;
this.butSomeText.Text = "Some Text";
this.butSomeText.Click += new System.EventHandler(this.butSomeText_Click);
//
// butSomeMoreText
//
this.butSomeMoreText.Location = new System.Drawing.Point(168, 240);
this.butSomeMoreText.Name = "butSomeMoreText";
this.butSomeMoreText.Size = new System.Drawing.Size(104, 23);
this.butSomeMoreText.TabIndex = 2;
this.butSomeMoreText.Text = "Some More Text";
this.butSomeMoreText.Click += new System.EventHandler(this.butSomeMoreText_Click);
//
// TextReader
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.butSomeMoreText,
this.butSomeText,
this.rtbText});
this.Name = "TextReader";
this.Text = "TextReader";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new TextReader());
}
private void butSomeText_Click(object sender, System.EventArgs e)
{
Class1 c = new Class1();
rtbText.Text = c.ReadText();
}
private void butSomeMoreText_Click(object sender, System.EventArgs e)
{
Class1 c = new Class1();
rtbText.Text = c.ReadMoreText();
}
}
/// <summary>
/// Summary description for Class1.
/// </summary>
public class Class1
{
public Class1()
{
//
// TODO: Add constructor logic here
//
}
public string ReadText()
{
//FileStream fs = File.OpenRead("C:\Documents and Settings\richard.conway\My Documents\Visual Studio Projects\TextReader\bin\Debug\readtext.rtf")
FileStream f = File.OpenRead(System.Environment.CurrentDirectory+"\\readtext.rtf");
StreamReader s = new StreamReader(f);
return s.ReadToEnd();
}
public string ReadMoreText()
{
FileStream f = File.OpenRead(System.Environment.CurrentDirectory+"\\readmoretext.rtf");
StreamReader s = new StreamReader(f);
return s.ReadToEnd();
}
}
}
TextReader.zip( 2 k)Related examples in the same category