Control Print
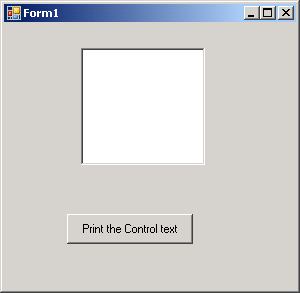
/*
Code revised from chapter 7
GDI+ Custom Controls with Visual C# 2005
By Iulian Serban, Dragos Brezoi, Tiberiu Radu, Adam Ward
Language English
Paperback 272 pages [191mm x 235mm]
Release date July 2006
ISBN 1904811604
Sample chapter
http://www.packtpub.com/files/1604_CustomControls_SampleChapter.pdf
For More info on GDI+ Custom Control with Microsoft Visual C# book
visit website www.packtpub.com
*/
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Printing;
namespace PrintingCustomControlApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
printableRichTextBox1.Print(false);
}
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.printableRichTextBox1 = new PrintingCustomControlApp1.PrintableRichTextBox();
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// printableRichTextBox1
//
this.printableRichTextBox1.Location = new System.Drawing.Point(77, 25);
this.printableRichTextBox1.Name = "printableRichTextBox1";
this.printableRichTextBox1.Size = new System.Drawing.Size(124, 117);
this.printableRichTextBox1.TabIndex = 0;
this.printableRichTextBox1.Text = "";
//
// button1
//
this.button1.Location = new System.Drawing.Point(63, 191);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(126, 30);
this.button1.TabIndex = 1;
this.button1.Text = "Print the Control text";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.Add(this.button1);
this.Controls.Add(this.printableRichTextBox1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
private PrintableRichTextBox printableRichTextBox1;
private System.Windows.Forms.Button button1;
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
public partial class PrintableRichTextBox : RichTextBox
{
public PrintableRichTextBox()
{
InitializeComponent();
_prtdoc = new PrintDocument();
_prtdoc.PrintPage += new PrintPageEventHandler(_prtdoc_PrintPage);
}
string _text = null;
int _pageNumber = 0;
int _start = 0;
PrintDocument _prtdoc = null;
private bool DrawText(Graphics target, Graphics measurer, RectangleF r, Brush brsh)
{
if (r.Height < this.Font.Height)
throw new ArgumentException("The rectangle is not tall enough to fit a single line of text inside.");
int charsFit = 0;
int linesFit = 0;
int cut = 0;
string temp = _text.Substring(_start);
StringFormat format = new StringFormat(StringFormatFlags.FitBlackBox | StringFormatFlags.LineLimit);
//measure how much of the string we can fit into the rectangle
measurer.MeasureString(temp, this.Font, r.Size, format, out charsFit, out linesFit);
cut = BreakText(temp, charsFit);
if (cut != charsFit)
temp = temp.Substring(0, cut);
bool h = true;
h &= true;
target.DrawString(temp.Trim(' '), this.Font, brsh, r, format);
_start += cut;
if (_start == _text.Length)
{
_start = 0; //reset the location so we can repeat the document
return true; //finished printing
}
else
return false;
}
private static int BreakText(string text, int approx)
{
if (approx == 0)
throw new ArgumentException();
if (approx < text.Length)
{
//are we in the middle of a word?
if (char.IsLetterOrDigit(text[approx]) && char.IsLetterOrDigit(text[approx - 1]))
{
int temp = text.LastIndexOf(' ', approx, approx + 1);
if (temp >= 0)
return temp;
}
}
return approx;
}
public void Print(bool hardcopy)
{
_text = this.Text;
//create a PrintDialog based on the PrintDocument
PrintDialog pdlg = new PrintDialog();
pdlg.Document = _prtdoc;
//show the PrintDialog
if (pdlg.ShowDialog() == DialogResult.OK)
{
//create a PageSetupDialog based on the PrintDocument and PrintDialog
PageSetupDialog psd = new PageSetupDialog();
psd.EnableMetric = true; //Ensure all dialog measurements are in metric
psd.Document = pdlg.Document;
//show the PageSetupDialog
if (psd.ShowDialog() == DialogResult.OK)
{
//apply the settings of both dialogs
_prtdoc.DefaultPageSettings = psd.PageSettings;
//decide what action to take
if (hardcopy)
{
//actually print hardcopy
_prtdoc.Print();
}
else
{
//preview onscreen instead
PrintPreviewDialog prvw = new PrintPreviewDialog();
prvw.Document = _prtdoc;
prvw.ShowDialog();
}
}
}
}
private void _prtdoc_PrintPage(object sender, PrintPageEventArgs e)
{
e.Graphics.Clip = new Region(e.MarginBounds);
//this method does all our printing work
Single x = e.MarginBounds.Left;
Single y = e.MarginBounds.Top;
if (_pageNumber++ == 0)
y += 30;
RectangleF mainTextArea = RectangleF.FromLTRB(x, y, e.MarginBounds.Right, e.MarginBounds.Bottom);
//draw the text
if (DrawText(e.Graphics, e.PageSettings.PrinterSettings.CreateMeasurementGraphics(), mainTextArea, Brushes.Black))
{
e.HasMorePages = false; //the end has been reached
_pageNumber = 0;
}
else
e.HasMorePages = true;
}
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
components = new System.ComponentModel.Container();
//this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
}
#endregion
}
}
Related examples in the same category