Date Time Picker 2
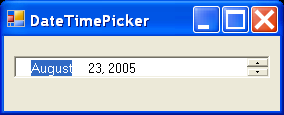
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace DateTimePicker
{
/// <summary>
/// Summary description for DateTimePicker2.
/// </summary>
public class DateTimePicker2 : System.Windows.Forms.Form
{
private System.Windows.Forms.DateTimePicker dateTimePicker1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public DateTimePicker2()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.dateTimePicker1 = new System.Windows.Forms.DateTimePicker();
this.SuspendLayout();
//
// dateTimePicker1
//
this.dateTimePicker1.Location = new System.Drawing.Point(8, 16);
this.dateTimePicker1.Name = "dateTimePicker1";
this.dateTimePicker1.RightToLeft = System.Windows.Forms.RightToLeft.No;
this.dateTimePicker1.ShowUpDown = true;
this.dateTimePicker1.TabIndex = 0;
//
// DateTimePicker2
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(216, 61);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.dateTimePicker1});
this.Name = "DateTimePicker2";
this.Text = "DateTimePicker";
this.Load += new System.EventHandler(this.DateTimePicker2_Load);
this.ResumeLayout(false);
}
#endregion
private void DateTimePicker2_Load(object sender, System.EventArgs e)
{
}
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new DateTimePicker2());
}
}
}
Related examples in the same category