Help icon
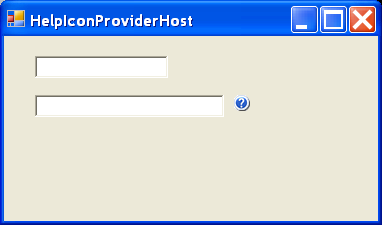
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace ExtenderProviderHost
{
/// <summary>
/// Summary description for HelpIconProviderHost.
/// </summary>
public class HelpIconProviderHost : System.Windows.Forms.Form
{
internal System.Windows.Forms.TextBox TextBox2;
internal System.Windows.Forms.TextBox TextBox1;
private ExtenderProviderControls.HelpIconProvider helpIconProvider1;
private System.ComponentModel.IContainer components;
public HelpIconProviderHost()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.TextBox2 = new System.Windows.Forms.TextBox();
this.TextBox1 = new System.Windows.Forms.TextBox();
this.helpIconProvider1 = new ExtenderProviderControls.HelpIconProvider(this.components);
this.SuspendLayout();
//
// TextBox2
//
this.TextBox2.Location = new System.Drawing.Point(24, 48);
this.TextBox2.Name = "TextBox2";
this.TextBox2.Size = new System.Drawing.Size(148, 20);
this.TextBox2.TabIndex = 3;
this.TextBox2.Text = "";
//
// TextBox1
//
this.TextBox1.Location = new System.Drawing.Point(24, 16);
this.TextBox1.Name = "TextBox1";
this.TextBox1.Size = new System.Drawing.Size(104, 20);
this.TextBox1.TabIndex = 2;
this.TextBox1.Text = "";
//
// helpIconProvider1
//
this.helpIconProvider1.HelpFile = null;
//
// HelpIconProviderHost
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 150);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.TextBox2,
this.TextBox1});
this.Name = "HelpIconProviderHost";
this.Text = "HelpIconProviderHost";
this.Load += new System.EventHandler(this.HelpIconProviderHost_Load);
this.ResumeLayout(false);
}
#endregion
private void HelpIconProviderHost_Load(object sender, System.EventArgs e)
{
helpIconProvider1.HelpFile = "myhelp.hlp";
helpIconProvider1.SetHelpID(TextBox1, "10001");
helpIconProvider1.SetHelpID(TextBox2, "10002");
helpIconProvider1.SetHelpID(TextBox1,"");
}
static void Main()
{
Application.Run(new HelpIconProviderHost());
}
}
}
//=============================================================
//=============================================================
using System;
using System.Windows.Forms;
using System.ComponentModel;
using System.Collections;
using System.Drawing;
namespace ExtenderProviderControls
{
[ProvideProperty("HelpID", typeof(string))]
public class HelpIconProvider : Component, IExtenderProvider
{
private System.ComponentModel.Container components = null;
public HelpIconProvider(System.ComponentModel.IContainer container)
{
/// <summary>
/// Required for Windows.Forms Class Composition Designer support
/// </summary>
container.Add(this);
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
public HelpIconProvider()
{
/// <summary>
/// Required for Windows.Forms Class Composition Designer support
/// </summary>
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(HelpIconProvider));
this.pictureBox1 = new System.Windows.Forms.PictureBox();
//
// pictureBox1
//
this.pictureBox1.Image = ((System.Drawing.Bitmap)(resources.GetObject("pictureBox1.Image")));
this.pictureBox1.Location = new System.Drawing.Point(17, 17);
this.pictureBox1.Name = "pictureBox1";
this.pictureBox1.TabIndex = 0;
this.pictureBox1.TabStop = false;
}
#endregion
private Hashtable contextID = new Hashtable();
private Hashtable pictures = new Hashtable();
private System.Windows.Forms.PictureBox pictureBox1;
private string helpFile;
public bool CanExtend(object extendee)
{
if (extendee.GetType() == typeof(Control))
{
// Ensure the control is attached to a form.
if (((Control)extendee).FindForm() == null)
{
return false;
}
else
{
return true;
}
}
else
{
return false;
}
}
public string HelpFile
{
get
{
return helpFile;
}
set
{
helpFile = value;
}
}
public void SetHelpID(object extendee, string value)
{
Control ctrl = (Control)extendee;
// Specifying an empty value removes the extension.
if (value == "")
{
contextID.Remove(extendee);
// Remove the picture.
PictureBox pic = (PictureBox)pictures[extendee];
pic.DoubleClick -= new EventHandler(PicDoubleClick);
pic.Parent.Controls.Remove(pic);
pictures.Remove(extendee);
}
else
{
contextID[extendee] = value;
// Create new icon.
PictureBox pic = new PictureBox();
pic.Image = pictureBox1.Image;
// Store a reference to the related control in the PictureBox.
pic.Tag = extendee;
pic.Size = new Size(16, 16);
pic.Location = new Point(ctrl.Right + 10, ctrl.Top);
ctrl.Parent.Controls.Add(pic);
// Register for DoubleClick event.
pic.DoubleClick += new EventHandler(PicDoubleClick);
// Store a reference to the help icon so we can remove it later.
pictures[extendee] = pic;
}
}
public string GetHelpID(object extendee)
{
if (contextID[extendee] != null)
{
return contextID[extendee].ToString();
}
else
{
return String.Empty;
}
}
public void PicDoubleClick(object sender, EventArgs e)
{
// Invoke help for control.
Control ctrlRelated = (Control)((Control)sender).Tag;
// Debug message.
MessageBox.Show("Help triggered.");
Help.ShowHelp(ctrlRelated, helpFile, HelpNavigator.Topic,
contextID[ctrlRelated].ToString());
}
}
}
HelpIconProviderHost.zip( 72 k)Related examples in the same category