Numeric value based Up Down (Spinner)
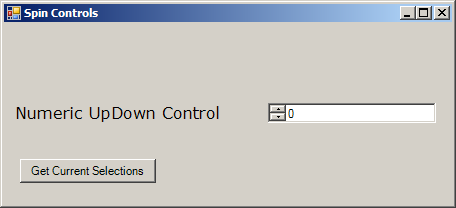
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class UpDownForm : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblCurrSel;
private System.Windows.Forms.Button btnGetSelections;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.NumericUpDown numericUpDown;
public UpDownForm()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label ();
this.numericUpDown = new System.Windows.Forms.NumericUpDown ();
this.btnGetSelections = new System.Windows.Forms.Button ();
this.lblCurrSel = new System.Windows.Forms.Label ();
numericUpDown.BeginInit ();
label1.Location = new System.Drawing.Point (8, 80);
label1.Text = "Numeric UpDown Control";
label1.Size = new System.Drawing.Size (232, 32);
label1.Font = new System.Drawing.Font ("Verdana", 12);
label1.TabIndex = 3;
numericUpDown.Location = new System.Drawing.Point (264, 80);
numericUpDown.Maximum = new decimal (5000);
numericUpDown.Size = new System.Drawing.Size (168, 20);
numericUpDown.ThousandsSeparator = true;
numericUpDown.TabIndex = 1;
numericUpDown.UpDownAlign = System.Windows.Forms.LeftRightAlignment.Left;
numericUpDown.ValueChanged += new System.EventHandler (this.numericUpDown_ValueChanged);
btnGetSelections.Location = new System.Drawing.Point (16, 136);
btnGetSelections.Size = new System.Drawing.Size (136, 24);
btnGetSelections.TabIndex = 4;
btnGetSelections.Text = "Get Current Selections";
btnGetSelections.Click += new System.EventHandler (this.btnGetSelections_Click);
lblCurrSel.Location = new System.Drawing.Point (176, 120);
lblCurrSel.Size = new System.Drawing.Size (256, 48);
this.Text = "Spin Controls";
this.AutoScaleBaseSize = new System.Drawing.Size (5, 13);
this.ClientSize = new System.Drawing.Size (448, 181);
this.Controls.Add (this.lblCurrSel);
this.Controls.Add (this.btnGetSelections);
this.Controls.Add (this.label1);
this.Controls.Add (this.numericUpDown);
numericUpDown.EndInit ();
}
static void Main()
{
Application.Run(new UpDownForm());
}
protected void numericUpDown_ValueChanged (object sender, System.EventArgs e)
{
this.Text = "You changed the numeric value...";
}
protected void btnGetSelections_Click (object sender, System.EventArgs e)
{
lblCurrSel.Text = "Number: "
+ numericUpDown.Value;
}
}
Related examples in the same category