PictureBox Demo
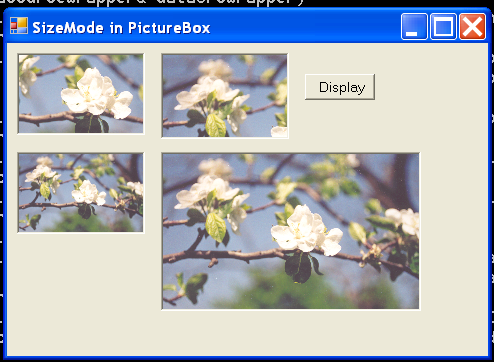
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace BictureBox
{
/// <summary>
/// Summary description for PictureBoxDemo.
/// </summary>
public class PictureBoxDemo : System.Windows.Forms.Form
{
private System.Windows.Forms.PictureBox pictureBox1;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.PictureBox pictureBox2;
private System.Windows.Forms.PictureBox pictureBox3;
private System.Windows.Forms.PictureBox pictureBox4;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public PictureBoxDemo()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
this.Text = "SizeMode in PictureBox";
this.button1.Text = "Display";
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.pictureBox1 = new System.Windows.Forms.PictureBox();
this.button1 = new System.Windows.Forms.Button();
this.pictureBox2 = new System.Windows.Forms.PictureBox();
this.pictureBox3 = new System.Windows.Forms.PictureBox();
this.pictureBox4 = new System.Windows.Forms.PictureBox();
this.SuspendLayout();
//
// pictureBox1
//
this.pictureBox1.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.pictureBox1.Location = new System.Drawing.Point(8, 8);
this.pictureBox1.Name = "pictureBox1";
this.pictureBox1.Size = new System.Drawing.Size(100, 70);
this.pictureBox1.TabIndex = 0;
this.pictureBox1.TabStop = false;
//
// button1
//
this.button1.Location = new System.Drawing.Point(232, 24);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(56, 23);
this.button1.TabIndex = 1;
this.button1.Text = "button1";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// pictureBox2
//
this.pictureBox2.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.pictureBox2.Location = new System.Drawing.Point(120, 8);
this.pictureBox2.Name = "pictureBox2";
this.pictureBox2.Size = new System.Drawing.Size(100, 70);
this.pictureBox2.TabIndex = 0;
this.pictureBox2.TabStop = false;
//
// pictureBox3
//
this.pictureBox3.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.pictureBox3.Location = new System.Drawing.Point(8, 88);
this.pictureBox3.Name = "pictureBox3";
this.pictureBox3.Size = new System.Drawing.Size(100, 70);
this.pictureBox3.TabIndex = 0;
this.pictureBox3.TabStop = false;
//
// pictureBox4
//
this.pictureBox4.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.pictureBox4.Location = new System.Drawing.Point(120, 88);
this.pictureBox4.Name = "pictureBox4";
this.pictureBox4.Size = new System.Drawing.Size(100, 70);
this.pictureBox4.TabIndex = 0;
this.pictureBox4.TabStop = false;
//
// PictureBoxDemo
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(376, 254);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button1,
this.pictureBox2,
this.pictureBox3,
this.pictureBox4,
this.pictureBox1});
this.Name = "PictureBoxDemo";
this.Text = "PictureBoxDemo";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new PictureBoxDemo());
}
private void button1_Click(object sender, System.EventArgs e)
{
SetPictureBoxSizeMode();
}
private void SetPictureBoxSizeMode()
{
string path = @"Dobos3.BMP"; // Change the path if needed.
pictureBox1.SizeMode = PictureBoxSizeMode.CenterImage;
pictureBox1.Image = Image.FromFile(path);
pictureBox2.SizeMode = PictureBoxSizeMode.Normal;
pictureBox2.Image = Image.FromFile(path);
pictureBox3.SizeMode = PictureBoxSizeMode.StretchImage;
pictureBox3.Image = Image.FromFile(path);
pictureBox4.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox4.Image = Image.FromFile(path);
}
}
}
P17_PictureBox.zip( 103 k)Related examples in the same category