RadioButton on a form
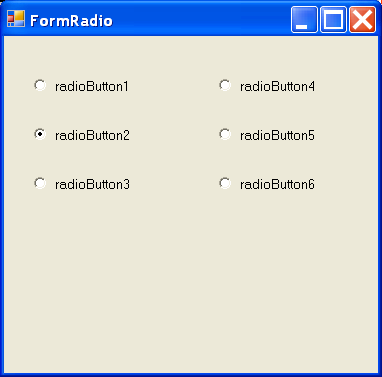
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Radio
{
/// <summary>
/// Summary description for FormRadio.
/// </summary>
public class FormRadio : System.Windows.Forms.Form
{
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.RadioButton radioButton2;
private System.Windows.Forms.RadioButton radioButton1;
private System.Windows.Forms.RadioButton radioButton3;
private System.Windows.Forms.RadioButton radioButton5;
private System.Windows.Forms.RadioButton radioButton4;
private System.Windows.Forms.Panel panel2;
private System.Windows.Forms.RadioButton radioButton6;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public FormRadio()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.panel1 = new System.Windows.Forms.Panel();
this.radioButton2 = new System.Windows.Forms.RadioButton();
this.radioButton1 = new System.Windows.Forms.RadioButton();
this.radioButton3 = new System.Windows.Forms.RadioButton();
this.radioButton5 = new System.Windows.Forms.RadioButton();
this.radioButton4 = new System.Windows.Forms.RadioButton();
this.panel2 = new System.Windows.Forms.Panel();
this.radioButton6 = new System.Windows.Forms.RadioButton();
this.panel1.SuspendLayout();
this.panel2.SuspendLayout();
this.SuspendLayout();
//
// panel1
//
this.panel1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.radioButton2,
this.radioButton1,
this.radioButton3});
this.panel1.Location = new System.Drawing.Point(8, 16);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(136, 136);
this.panel1.TabIndex = 1;
//
// radioButton2
//
this.radioButton2.Location = new System.Drawing.Point(16, 56);
this.radioButton2.Name = "radioButton2";
this.radioButton2.Size = new System.Drawing.Size(96, 16);
this.radioButton2.TabIndex = 0;
this.radioButton2.Text = "radioButton2";
//
// radioButton1
//
this.radioButton1.Location = new System.Drawing.Point(16, 16);
this.radioButton1.Name = "radioButton1";
this.radioButton1.Size = new System.Drawing.Size(96, 16);
this.radioButton1.TabIndex = 0;
this.radioButton1.Text = "radioButton1";
//
// radioButton3
//
this.radioButton3.Location = new System.Drawing.Point(16, 96);
this.radioButton3.Name = "radioButton3";
this.radioButton3.Size = new System.Drawing.Size(96, 16);
this.radioButton3.TabIndex = 0;
this.radioButton3.Text = "radioButton3";
//
// radioButton5
//
this.radioButton5.Location = new System.Drawing.Point(16, 56);
this.radioButton5.Name = "radioButton5";
this.radioButton5.Size = new System.Drawing.Size(96, 16);
this.radioButton5.TabIndex = 0;
this.radioButton5.Text = "radioButton5";
//
// radioButton4
//
this.radioButton4.Location = new System.Drawing.Point(16, 16);
this.radioButton4.Name = "radioButton4";
this.radioButton4.Size = new System.Drawing.Size(96, 16);
this.radioButton4.TabIndex = 0;
this.radioButton4.Text = "radioButton4";
//
// panel2
//
this.panel2.Controls.AddRange(new System.Windows.Forms.Control[] {
this.radioButton6,
this.radioButton4,
this.radioButton5});
this.panel2.Location = new System.Drawing.Point(152, 16);
this.panel2.Name = "panel2";
this.panel2.Size = new System.Drawing.Size(136, 136);
this.panel2.TabIndex = 2;
//
// radioButton6
//
this.radioButton6.Location = new System.Drawing.Point(16, 96);
this.radioButton6.Name = "radioButton6";
this.radioButton6.Size = new System.Drawing.Size(96, 16);
this.radioButton6.TabIndex = 0;
this.radioButton6.Text = "radioButton6";
//
// FormRadio
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.panel1,
this.panel2});
this.Name = "FormRadio";
this.Text = "FormRadio";
this.panel1.ResumeLayout(false);
this.panel2.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new FormRadio());
}
}
}
Related examples in the same category