Scrollable Form
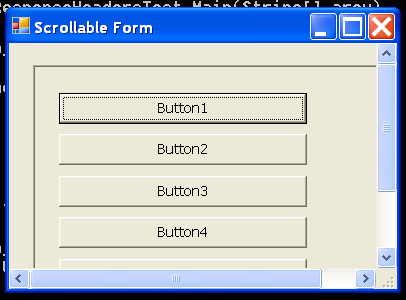
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace UnsualScrolling
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class ScrollableForm : System.Windows.Forms.Form
{
internal System.Windows.Forms.Panel Panel1;
internal System.Windows.Forms.Button Button6;
internal System.Windows.Forms.Button Button5;
internal System.Windows.Forms.Button Button4;
internal System.Windows.Forms.Button Button3;
internal System.Windows.Forms.Button Button2;
internal System.Windows.Forms.Button Button1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public ScrollableForm()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Panel1 = new System.Windows.Forms.Panel();
this.Button6 = new System.Windows.Forms.Button();
this.Button5 = new System.Windows.Forms.Button();
this.Button4 = new System.Windows.Forms.Button();
this.Button3 = new System.Windows.Forms.Button();
this.Button2 = new System.Windows.Forms.Button();
this.Button1 = new System.Windows.Forms.Button();
this.Panel1.SuspendLayout();
this.SuspendLayout();
//
// Panel1
//
this.Panel1.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.Panel1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.Button6,
this.Button5,
this.Button4,
this.Button3,
this.Button2,
this.Button1});
this.Panel1.Location = new System.Drawing.Point(16, 17);
this.Panel1.Name = "Panel1";
this.Panel1.Size = new System.Drawing.Size(260, 232);
this.Panel1.TabIndex = 1;
//
// Button6
//
this.Button6.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button6.Location = new System.Drawing.Point(16, 180);
this.Button6.Name = "Button6";
this.Button6.Size = new System.Drawing.Size(168, 24);
this.Button6.TabIndex = 5;
this.Button6.Text = "Button6";
//
// Button5
//
this.Button5.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button5.Location = new System.Drawing.Point(16, 148);
this.Button5.Name = "Button5";
this.Button5.Size = new System.Drawing.Size(168, 24);
this.Button5.TabIndex = 4;
this.Button5.Text = "Button5";
//
// Button4
//
this.Button4.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button4.Location = new System.Drawing.Point(16, 116);
this.Button4.Name = "Button4";
this.Button4.Size = new System.Drawing.Size(168, 24);
this.Button4.TabIndex = 3;
this.Button4.Text = "Button4";
//
// Button3
//
this.Button3.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button3.Location = new System.Drawing.Point(16, 84);
this.Button3.Name = "Button3";
this.Button3.Size = new System.Drawing.Size(168, 24);
this.Button3.TabIndex = 2;
this.Button3.Text = "Button3";
//
// Button2
//
this.Button2.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button2.Location = new System.Drawing.Point(16, 52);
this.Button2.Name = "Button2";
this.Button2.Size = new System.Drawing.Size(168, 24);
this.Button2.TabIndex = 1;
this.Button2.Text = "Button2";
//
// Button1
//
this.Button1.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button1.Location = new System.Drawing.Point(16, 20);
this.Button1.Name = "Button1";
this.Button1.Size = new System.Drawing.Size(168, 24);
this.Button1.TabIndex = 0;
this.Button1.Text = "Button1";
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.AutoScroll = true;
this.ClientSize = new System.Drawing.Size(211, 169);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.Panel1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Name = "Form1";
this.Text = "Scrollable Form";
this.Panel1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new ScrollableForm());
}
}
}
Related examples in the same category