Demonstrates combining and removing delegates to create new delegates
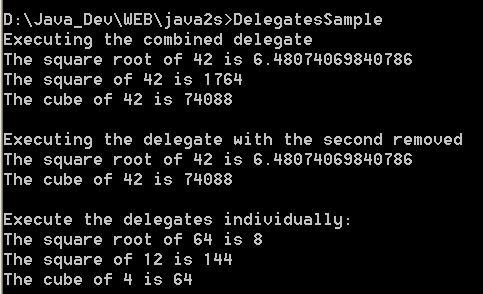
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// DlgOps.cs -- demonstrates combining and removing delegates to create
// new delegates.
//
// Compile this program with the following command line:
// C:>csc DlgOps.cs
using System;
namespace nsDelegates
{
public class DelegatesSample
{
public delegate void MathHandler (double val);
static public void Main ()
{
DelegatesSample main = new DelegatesSample ();
MathHandler dlg1, dlg2, dlg3;
dlg1 = new MathHandler (main.TheSquareRoot);
dlg2 = new MathHandler (main.TheSquare);
dlg3 = new MathHandler (main.TheCube);
// Combine the delegates so you can execute all three at one on one value
MathHandler dlgCombo = dlg1 + dlg2 + dlg3;
Console.WriteLine ("Executing the combined delegate");
dlgCombo (42);
// Now remove the second delegate
MathHandler dlgMinus = dlgCombo - dlg2;
Console.WriteLine ("\r\nExecuting the delegate with the second removed");
dlgMinus (42);
// Show that the individual delegates are stil available
// Execute the delegates one at a time using different values
Console.WriteLine ("\r\nExecute the delegates individually:");
dlg1 (64);
dlg2 (12);
dlg3 (4);
}
public void TheSquareRoot (double val)
{
Console.WriteLine ("The square root of " + val + " is " + Math.Sqrt (val));
}
public void TheSquare (double val)
{
Console.WriteLine ("The square of " + val + " is " + val * val);
}
public void TheCube (double val)
{
Console.WriteLine ("The cube of " + val + " is " + val * val * val);
}
}
}
Related examples in the same category