Demonstrates using as a statement
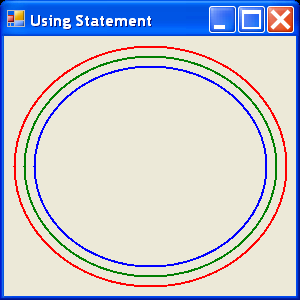
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// Using.cs -- Demonstrates using as a statement
//
// Compile this program with the following command line:
// C:>csc Using.cs
using System;
using System.Windows.Forms;
using System.Drawing;
using Pen = System.Drawing.Pen;
using PaintHandler = System.Windows.Forms.PaintEventHandler;
namespace nsForm
{
public class UsingForm : Form
{
public UsingForm ()
{
this.Text = "Using Statement";
this.Paint += new PaintHandler(this.OnPaint);
}
static public void Main ()
{
Application.Run(new UsingForm());
}
private Color [] clr = new Color []
{
Color.Red,
Color.Green,
Color.Blue
};
private void OnPaint (object obj, PaintEventArgs e)
{
Rectangle client = this.ClientRectangle;
int side = (client.Right - client.Left) / 3;
for (int x = 0; x < 3; ++x)
{
using (Pen pen = new Pen(clr[x], (float) 2.0))
{
client = Rectangle.Inflate (client, -10, -10);
e.Graphics.DrawEllipse (pen, client);
}
}
}
}
}
Related examples in the same category