Demonstrate the effects of pointer arithmethic
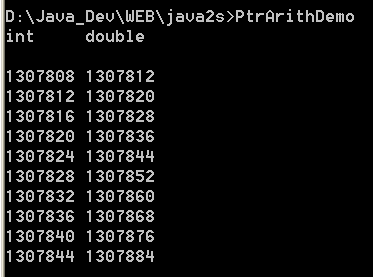
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate the effects of pointer arithmethic.
using System;
public class PtrArithDemo {
unsafe public static void Main() {
int x;
int i;
double d;
int* ip = &i;
double* fp = &d;
Console.WriteLine("int double\n");
for(x=0; x < 10; x++) {
Console.WriteLine((uint) (ip) + " " +
(uint) (fp));
ip++;
fp++;
}
}
}
Related examples in the same category