Objects can be passed to methods
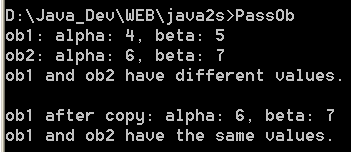
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Objects can be passed to methods.
using System;
class MyClass {
int alpha, beta;
public MyClass(int i, int j) {
alpha = i;
beta = j;
}
/* Return true if ob contains the same values
as the invoking object. */
public bool sameAs(MyClass ob) {
if((ob.alpha == alpha) & (ob.beta == beta))
return true;
else return false;
}
// Make a copy of ob.
public void copy(MyClass ob) {
alpha = ob.alpha;
beta = ob.beta;
}
public void show() {
Console.WriteLine("alpha: {0}, beta: {1}",
alpha, beta);
}
}
public class PassOb {
public static void Main() {
MyClass ob1 = new MyClass(4, 5);
MyClass ob2 = new MyClass(6, 7);
Console.Write("ob1: ");
ob1.show();
Console.Write("ob2: ");
ob2.show();
if(ob1.sameAs(ob2))
Console.WriteLine("ob1 and ob2 have the same values.");
else
Console.WriteLine("ob1 and ob2 have different values.");
Console.WriteLine();
// now, make ob1 a copy of ob2
ob1.copy(ob2);
Console.Write("ob1 after copy: ");
ob1.show();
if(ob1.sameAs(ob2))
Console.WriteLine("ob1 and ob2 have the same values.");
else
Console.WriteLine("ob1 and ob2 have different values.");
}
}
Related examples in the same category