Switch based console menu
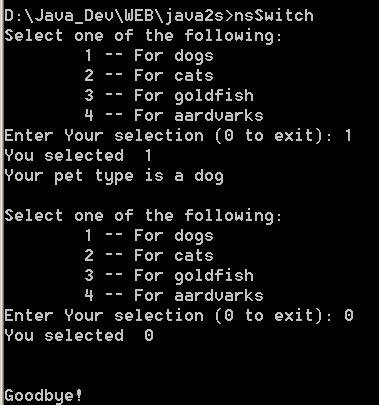
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
namespace nsSwitch
{
using System;
public class nsSwitch
{
static void Main ()
{
bool done = false;
do
{
clsAnimal dog = new clsAnimal (1);
clsAnimal cat = new clsAnimal (2);
clsAnimal goldfish = new clsAnimal (3);
clsAnimal aardvark = new clsAnimal (4);
Console.WriteLine ("Select one of the following:");
Console.WriteLine ("\t1 -- For dogs");
Console.WriteLine ("\t2 -- For cats");
Console.WriteLine ("\t3 -- For goldfish");
Console.WriteLine ("\t4 -- For aardvarks");
Console.Write ("Enter Your selection (0 to exit): ");
string strSelection = Console.ReadLine ();
int iSel;
try
{
iSel = int.Parse(strSelection);
}
catch (FormatException)
{
Console.WriteLine ("\r\nWhat?\r\n");
continue;
}
Console.WriteLine ("You selected " + iSel);
switch (iSel)
{
case 0:
done = true;
break;
case 1:
Console.WriteLine (dog);
break;
case 2:
Console.WriteLine (cat);
break;
case 3:
Console.WriteLine (goldfish);
break;
case 4:
Console.WriteLine (aardvark);
break;
default:
Console.WriteLine ("You selected an invalid number: {0}\r\n", iSel);
continue;
}
Console.WriteLine ();
} while (!done);
Console.WriteLine ("\nGoodbye!");
}
}
class clsAnimal
{
public clsAnimal (int Type)
{
PetType = Type;
}
private int Type;
public int PetType
{
get {return (Type);}
set {Type = value;}
}
public override string ToString()
{
switch (PetType)
{
default:
return ("Unknown pet");
case 1:
return ("Your pet type is a dog");
case 2:
return ("Your pet type is a cat");
case 3:
return ("Your pet type is a goldfish");
case 4:
return ("Your pet type is an aardvark");
}
}
}
}
Related examples in the same category