illustrates exception propagation with methods
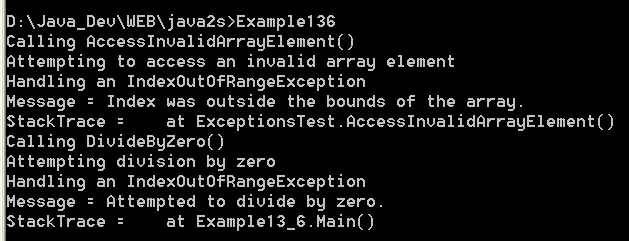
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example13_6.cs illustrates exception propagation
with methods
*/
using System;
// declare the ExceptionsTest class
class ExceptionsTest
{
public void AccessInvalidArrayElement()
{
int[] myArray = new int[2];
try
{
Console.WriteLine("Attempting to access an invalid array element");
myArray[2] = 1;
}
catch (IndexOutOfRangeException e)
{
Console.WriteLine("Handling an IndexOutOfRangeException");
Console.WriteLine("Message = " + e.Message);
Console.WriteLine("StackTrace = " + e.StackTrace);
}
}
public void DivideByZero()
{
int zero = 0;
Console.WriteLine("Attempting division by zero");
int myInt = 1 / zero;
}
}
public class Example13_6
{
public static void Main()
{
ExceptionsTest myExceptionsTest = new ExceptionsTest();
// call the AccessInvalidArrayElement() method,
// this method handles the exception locally
Console.WriteLine("Calling AccessInvalidArrayElement()");
myExceptionsTest.AccessInvalidArrayElement();
try
{
// call the DivideByZero() method,
// this method doesn't handle the exception locally and
// so it must be handled here
Console.WriteLine("Calling DivideByZero()");
myExceptionsTest.DivideByZero();
}
catch (DivideByZeroException e)
{
Console.WriteLine("Handling an IndexOutOfRangeException");
Console.WriteLine("Message = " + e.Message);
Console.WriteLine("StackTrace = " + e.StackTrace);
}
}
}
Related examples in the same category