An keypress event example
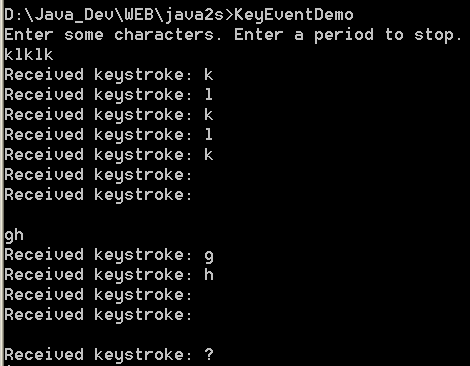
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// An keypress event example.
using System;
// Derive a custom EventArgs class that holds the key.
class KeyEventArgs : EventArgs {
public char ch;
}
// Declare a delegate for an event.
delegate void KeyHandler(object source, KeyEventArgs arg);
// Declare a key-press event class.
class KeyEvent {
public event KeyHandler KeyPress;
// This is called when a key is pressed.
public void OnKeyPress(char key) {
KeyEventArgs k = new KeyEventArgs();
if(KeyPress != null) {
k.ch = key;
KeyPress(this, k);
}
}
}
// A class that receives key-press notifications.
class ProcessKey {
public void keyhandler(object source, KeyEventArgs arg) {
Console.WriteLine("Received keystroke: " + arg.ch);
}
}
// Another class that receives key-press notifications.
class CountKeys {
public int count = 0;
public void keyhandler(object source, KeyEventArgs arg) {
count++;
}
}
// Demonstrate KeyEvent.
public class KeyEventDemo {
public static void Main() {
KeyEvent kevt = new KeyEvent();
ProcessKey pk = new ProcessKey();
CountKeys ck = new CountKeys();
char ch;
kevt.KeyPress += new KeyHandler(pk.keyhandler);
kevt.KeyPress += new KeyHandler(ck.keyhandler);
Console.WriteLine("Enter some characters. " +
"Enter a period to stop.");
do {
ch = (char) Console.Read();
kevt.OnKeyPress(ch);
} while(ch != '.');
Console.WriteLine(ck.count + " keys pressed.");
}
}
Related examples in the same category