Demonstates starting and waiting on a thread
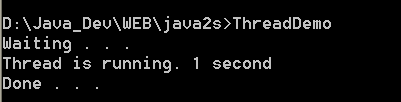
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// Thread.cs -- Demonstates starting and waiting on a thread
//
// Compile this program with the following command line:
// C:>csc Thread.cs
using System;
using System.Windows.Forms;
using System.Threading;
namespace nsThreads
{
public class ThreadDemo
{
static public void Main ()
{
// Create the new thread object
Thread NewThread = new Thread (new ThreadStart (RunThread));
// Show a message box.
MessageBox.Show ("Click OK to start the thread", "Thread Start");
// Start the new thread.
NewThread.Start ();
// Inform everybody that the main thread is waiting
Console.WriteLine ("Waiting . . .");
// Wait for NewThread to terminate.
NewThread.Join ();
// And it's done.
Console.WriteLine ("\r\nDone . . .");
}
// Method to assign to the new thread as its start method
static public void RunThread ()
{
// Sleep for a second, print and message and repeat.
for (int x = 5; x > 0; --x)
{
Thread.Sleep (1000);
Console.Write ("Thread is running. {0} second{1} \r",
x, x > 1 ? "s" : "");
}
// The thread will terminate at this point. It will not return to
// the method that started it.
}
}
}
Related examples in the same category