Change the margins of an element that is within a Grid by XAML and programmatic code
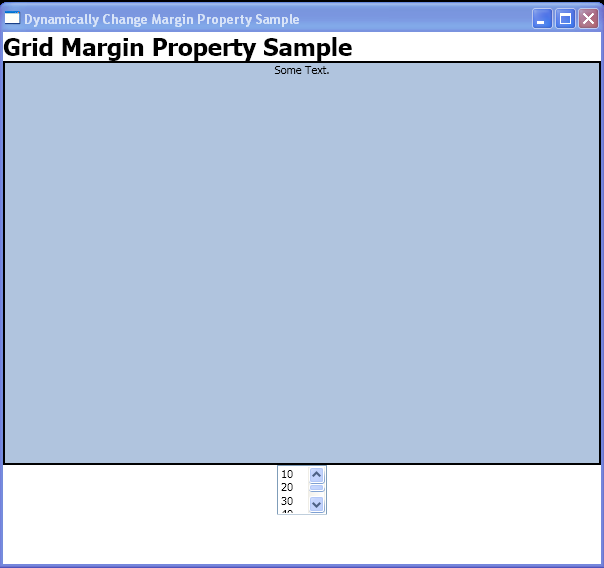
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="WpfApplication1.Window1"
Title="Dynamically Change Margin Property Sample">
<DockPanel Background="White">
<TextBlock DockPanel.Dock="Top" FontSize="24" FontWeight="Bold">Grid Margin Property Sample</TextBlock>
<Border Border.Background="LightSteelBlue" Border.BorderThickness="2" Border.BorderBrush="Black" DockPanel.Dock="Top">
<Grid Name="grid1" Height="400">
<Grid.ColumnDefinitions>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition/>
</Grid.RowDefinitions>
<TextBlock Name="text1" HorizontalAlignment="Center" Grid.Column="0" Grid.Row="0">Some Text.</TextBlock>
</Grid>
</Border>
<Grid HorizontalAlignment="Center" Width="300" DockPanel.Dock="Top">
<Grid.RowDefinitions>
<RowDefinition/>
<RowDefinition/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<ListBox Grid.Row="0" Grid.Column="1" Width="50" Height="50" VerticalAlignment="Top" SelectionChanged="ChangeMargin">
<ListBoxItem>10</ListBoxItem>
<ListBoxItem>20</ListBoxItem>
<ListBoxItem>30</ListBoxItem>
<ListBoxItem>40</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>60</ListBoxItem>
<ListBoxItem>70</ListBoxItem>
<ListBoxItem>80</ListBoxItem>
<ListBoxItem>90</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
</ListBox>
</Grid>
</DockPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Navigation;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public void ChangeMargin(object sender, SelectionChangedEventArgs args)
{
ListBoxItem li = ((sender as ListBox).SelectedItem as ListBoxItem);
ThicknessConverter myThicknessConverter = new ThicknessConverter();
Thickness th1 = (Thickness)myThicknessConverter.ConvertFromString(li.Content.ToString());
text1.Margin = th1;
String st1 = (String)myThicknessConverter.ConvertToString(text1.Margin);
Console.WriteLine("Margin: " + st1);
}
}
}
Related examples in the same category