Load or Save the Content of a RichTextBox
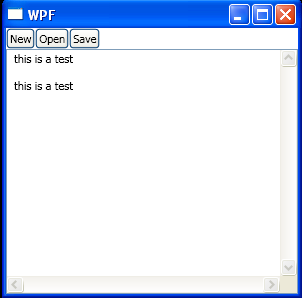
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<DockPanel>
<StackPanel DockPanel.Dock="Top" Orientation="Horizontal">
<Button Content="_New" Name="btnNew" Click="btnNew_Click" />
<Button Content="_Open" Name="btnOpen" Click="btnOpen_Click" />
<Button Content="_Save" Name="btnSave" Click="btnSave_Click" />
</StackPanel>
<RichTextBox DockPanel.Dock="Bottom" Name="rtbTextBox1"
HorizontalScrollBarVisibility="Visible"
VerticalScrollBarVisibility="Visible">
<FlowDocument>
<Paragraph>this is a test</Paragraph>
<Paragraph>this is a test</Paragraph>
</FlowDocument>
</RichTextBox>
</DockPanel>
</Window>
//File:Window.xaml.cs
using Microsoft.Win32;
using System;
using System.IO;
using System.Windows;
using System.Windows.Documents;
using System.Windows.Markup;
namespace WpfApplication1
{
public partial class Window1 : Window
{
private String currentFileName = String.Empty;
public Window1()
{
InitializeComponent();
}
private void btnOpen_Click(object sender, RoutedEventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.FileName = currentFileName;
dialog.Filter = "XAML Files (*.xaml)|*.xaml";
if (dialog.ShowDialog() == true)
{
currentFileName = dialog.FileName;
{
using (FileStream stream = File.Open(currentFileName, FileMode.Open))
{
FlowDocument doc = XamlReader.Load(stream) as FlowDocument;
if (doc == null)
{
MessageBox.Show("Could not load document.", Title);
}
else
{
rtbTextBox1.Document = doc;
}
}
}
}
}
private void btnNew_Click(object sender, RoutedEventArgs e)
{
rtbTextBox1.Document = new FlowDocument();
currentFileName = String.Empty;
}
private void btnSave_Click(object sender, RoutedEventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
dialog.FileName = currentFileName;
dialog.Filter = "XAML Files (*.xaml)|*.xaml";
if (dialog.ShowDialog() == true)
{
currentFileName = dialog.FileName;
using (FileStream stream = File.Open(currentFileName, FileMode.Create))
{
XamlWriter.Save(rtbTextBox1.Document, stream);
}
}
}
}
}
Related examples in the same category