Panel is setting the data context to the scrollbar object
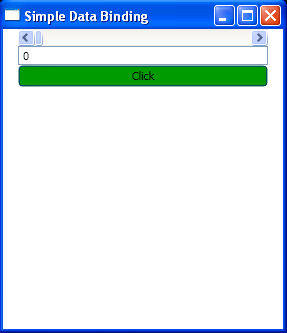
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:myConverters ="clr-namespace:WpfApplication1"
Title="Simple Data Binding" Height="334" Width="288"
WindowStartupLocation="CenterScreen"
>
<Window.Resources>
<myConverters:MyDoubleConverter x:Key="DoubleConverter"/>
<myConverters:MyColorConverter x:Key="ColorConverter"/>
</Window.Resources>
<StackPanel Width="250" DataContext = "{Binding ElementName=mySB}">
<ScrollBar Orientation="Horizontal" Name="mySB" Maximum = "100" LargeChange="1" SmallChange="1"/>
<TextBox Name="txtThumbValue" Text = "{Binding Path=Value, Converter={StaticResource DoubleConverter}}"/>
<Button Content="Click" FontSize = "{Binding Path=Value}" Background= "{Binding Path=Value, Converter={StaticResource ColorConverter}}"/>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Collections.Generic;
namespace WpfApplication1
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
}
class MyColorConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
double d = (double)value;
byte v = (byte)d;
Color color = new Color();
color.A = 255;
color.G = (byte) (155 + v);
return new SolidColorBrush(color);
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
return value;
}
}
class MyDoubleConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
double v = (double)value;
return (int)v;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return value;
}
}
}
Related examples in the same category