TextBox: set text, select all, clear, prepend, insert, append, cut, paste, undo
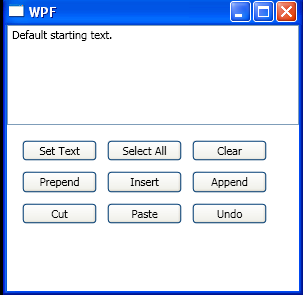
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<TextBox AcceptsReturn="True" Height="100" IsReadOnly="True"
Name="textBox1" TextAlignment="Left" TextWrapping="Wrap"
VerticalScrollBarVisibility="Auto">
Default starting text.
</TextBox>
<WrapPanel Margin="10">
<Button Margin="5" Name="textButton" Width="75" Click="TextButton_Click">Set Text</Button>
<Button Margin="5" Name="selectAllButton" Width="75" Click="SelectAllButton_Click">Select All</Button>
<Button Margin="5" Name="clearButton" Width="75" Click="ClearButton_Click">Clear</Button>
<Button Margin="5" Name="prependButton" Width="75" Click="PrependButton_Click">Prepend</Button>
<Button Margin="5" Name="insertButton" Width="75" Click="InsertButton_Click">Insert</Button>
<Button Margin="5" Name="appendButton" Width="75" Click="AppendButton_Click">Append</Button>
<Button Margin="5" Name="cutButton" Width="75" Click="CutButton_Click">Cut</Button>
<Button Margin="5" Name="pasteButton" Width="75" Click="PasteButton_Click">Paste</Button>
<Button Margin="5" Name="undoButton" Width="75" Click="UndoButton_Click">Undo</Button>
</WrapPanel>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void AppendButton_Click(object sender, RoutedEventArgs e)
{
textBox1.AppendText("text");
}
private void ClearButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Clear();
}
private void CutButton_Click(object sender, RoutedEventArgs e)
{
if (textBox1.SelectionLength == 0)
{
MessageBox.Show("Select text to cut first.", Title);
}
else
{
MessageBox.Show("Cut: " + textBox1.SelectedText, Title);
textBox1.Cut();
}
}
private void InsertButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Text = textBox1.Text.Insert(textBox1.CaretIndex, "text");
}
private void PasteButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Paste();
}
private void PrependButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Text = textBox1.Text.Insert(0, "Prepend");
}
private void SelectAllButton_Click(object sender, RoutedEventArgs e)
{
textBox1.SelectAll();
textBox1.Focus();
}
private void TextButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Text = "new value";
}
private void UndoButton_Click(object sender, RoutedEventArgs e)
{
textBox1.Undo();
}
}
}
Related examples in the same category