ToolTip With Binding
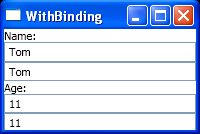
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1"
Title="WithBinding" Height="135" Width="200">
<Window.Resources>
<local:Employee x:Key="Tom" Name="Tom" Age="11" />
<local:AgeToForegroundConverter x:Key="ageConverter" />
<local:Base16Converter x:Key="Base16Converter" />
</Window.Resources>
<StackPanel DataContext="{StaticResource Tom}">
<TextBlock>Name:</TextBlock>
<TextBox Text="{Binding Path=Name}" />
<TextBox Text="{Binding Path=Name, Source={StaticResource Tom}}" />
<TextBlock>Age:</TextBlock>
<TextBox>
<TextBox.Text>
<Binding Path="Age" />
</TextBox.Text>
</TextBox>
<TextBox Name="ageTextBox" Text="{Binding Path=Age}"
Foreground="{Binding Path=Age, Converter={StaticResource ageConverter}}" />
<TextBox Text="{Binding Path=Age, Converter={StaticResource Base16Converter}}"
Foreground="{Binding Path=Age, Converter={StaticResource ageConverter}}" />
<TextBox Name="ageTextBoxOneWay" Foreground="{Binding Path=Age, Mode=OneWay,Source={StaticResource Tom}, Converter={StaticResource ageConverter}}"
ToolTip="{Binding RelativeSource={RelativeSource Self}, Path=(Validation.Errors)[0].ErrorContent}">
<TextBox.Text>
<Binding Path="Age" UpdateSourceTrigger="PropertyChanged">
<Binding.ValidationRules>
<local:NumberRangeRule Min="0" Max="128" />
</Binding.ValidationRules>
</Binding>
</TextBox.Text>
</TextBox>
<Button Name="birthdayButton" Foreground="{Binding Path=Foreground, ElementName=ageTextBox}">Birthday</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
using System.Diagnostics;
namespace WpfApplication1 {
public class Employee : INotifyPropertyChanged {
public event PropertyChangedEventHandler PropertyChanged;
protected void Notify(string propName) {
if( this.PropertyChanged != null ) {
PropertyChanged(this, new PropertyChangedEventArgs(propName));
}
}
string name;
public string Name {
get { return this.name; }
set {
this.name = value;
Notify("Name");
}
}
int age;
public int Age {
get { return this.age; }
set {
this.age = value;
Notify("Age");
}
}
public Employee() { }
public Employee(string name, int age) {
this.name = name;
this.age = age;
}
}
public partial class Window1 : Window {
public Window1() {
InitializeComponent();
this.birthdayButton.Click += birthdayButton_Click;
}
void birthdayButton_Click(object sender, RoutedEventArgs e) {
Employee emp = (Employee)this.FindResource("Tom");
++emp.Age;
Console.WriteLine(emp.Name);
Console.WriteLine(emp.Age);
}
}
[ValueConversion(/* sourceType */ typeof(int), /* targetType */ typeof(Brush))]
public class AgeToForegroundConverter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
if( targetType != typeof(Brush) ) { return null; }
int age = int.Parse(value.ToString());
return (age > 60 ? Brushes.Red : Brushes.Black);
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
throw new NotImplementedException();
}
}
public class Base16Converter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
return ((int)value).ToString("x");
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
return int.Parse((string)value, System.Globalization.NumberStyles.HexNumber);
}
}
public class NumberRangeRule : ValidationRule {
int _min;
public int Min {
get { return _min; }
set { _min = value; }
}
int _max;
public int Max {
get { return _max; }
set { _max = value; }
}
public override ValidationResult Validate(object value, System.Globalization.CultureInfo cultureInfo) {
int number;
if( !int.TryParse((string)value, out number) ) {
return new ValidationResult(false, "Invalid number format");
}
if( number < _min || number > _max ) {
string s = string.Format("Number out of range ({0}-{1})", _min, _max);
return new ValidationResult(false, s);
}
return ValidationResult.ValidResult;
}
}
}
Related examples in the same category