Use RadioButton to control TextBox alignment
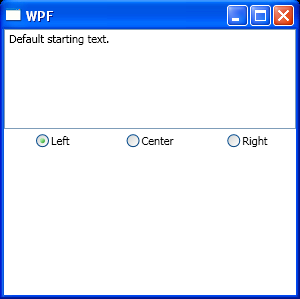
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<TextBox AcceptsReturn="True" Height="100" IsReadOnly="True"
Name="textBox1" TextAlignment="Left" TextWrapping="Wrap"
VerticalScrollBarVisibility="Auto">
Default starting text.
</TextBox>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<RadioButton Checked="AlignmentChecked" Grid.Column="0"
HorizontalAlignment="Center" IsChecked="True"
Margin="5" Name="leftAlignRadioButton">
Left</RadioButton>
<RadioButton Checked="AlignmentChecked" Grid.Column="1"
HorizontalAlignment="Center" Margin="5"
Name="centerAlignRadioButton">
Center</RadioButton>
<RadioButton Checked="AlignmentChecked" Grid.Column="2"
HorizontalAlignment="Center" Margin="5"
Name="rightAlignRadioButton">
Right</RadioButton>
</Grid>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void AlignmentChecked(object sender, RoutedEventArgs e)
{
RadioButton button = e.OriginalSource as RadioButton;
if (e.OriginalSource == leftAlignRadioButton)
{
textBox1.TextAlignment = TextAlignment.Left;
}
else if (e.OriginalSource == centerAlignRadioButton)
{
textBox1.TextAlignment = TextAlignment.Center;
}
else if (e.OriginalSource == rightAlignRadioButton)
{
textBox1.TextAlignment = TextAlignment.Right;
}
textBox1.Focus();
}
}
}
Related examples in the same category